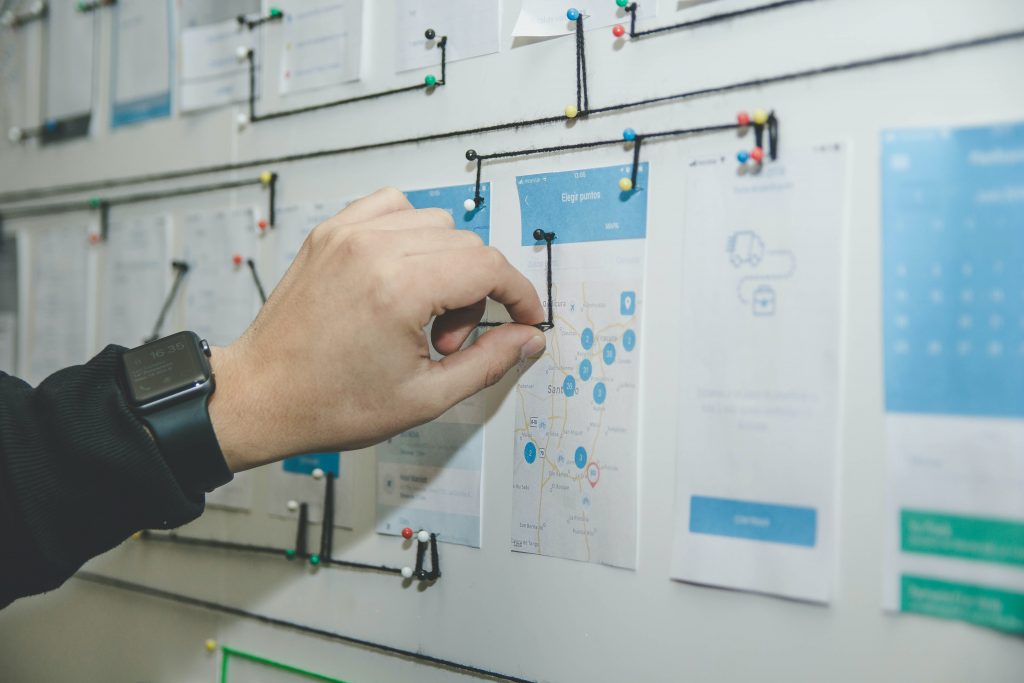
You might have already heard of the Drawer Menu here, but this time we’re going to utilize Material Design’s Navigation Drawer. It’s similar, but the Material Design has some extra and advanced features that you could utilize and switch from the standard drawer menu in flutter. The drawer menu is almost implemented in any app that exists today. That is why it’s important to know how adding a navigation drawer in flutter helps us to upgrade our apps that still use the standard drawer menu. Let’s get started!
Navigation Drawer Fundamentals In Flutter
Navigation drawers allow you to reach destinations in your app. Additionally, Navigation drawers allow access to locations as well as app functions such as account switching. They can be either permanently shown on the screen or controlled by a navigation menu icon.
Navigation Drawer Dos and Donts
Do:
- Use a navigation drawer for five or more primary destinations.
- When coupled with a bottom app bar scaled down to 62.5 percent, a navigation drawer sheet can open from the left side of the screen for left-to-right languages, or from the bottom of the screen for right-to-left languages.
- Navigation drawers can utilize text labels without icons.
- Keep text labels short and to the point, but truncate them if they go over the container width.
- When conventions exist, use familiar iconography.
- To separate groups of destinations, use full-width dividers.
- If access to account switching is critical, an account switcher can be put in the navigation drawer’s header section.
- If a navigation drawer takes up the entire vertical height of the page, a branding element or product name can be placed in the header space.
- A navigation menu icon always opens a modal drawer.
- Open bottom navigation drawers with a few objects to their full height.
- Adjust the bottom navigation drawer’s opening position such that the last list item in view is clipped by the screen’s bottom. This might alert viewers that there are other items to view.
- Allow scrolling of the contents of a bottom drawer when it is at full height.
Donts:
- If the app is set to a right-to-left language, do not open a navigation drawer from the right side of the screen.
- Label text should not be wrapped.
- Don’t reduce text size to fit a text label on a single line.
- Use different icons to represent distinct key destinations.
- Don’t use different icons for different destinations. All destinations should have icons, or none at all.
- Dividers should not be used to separate particular destinations.
- Place a branding element or product name in the header if a navigation drawer is clipped by a top app bar. In this scenario, the top app bar is a better location for that content.
- Don’t let a drawer’s height exceed its contents.
- In landscape mode, do not open a bottom drawer to half the screen height.
- When a bottom drawer isn’t at full-screen height, don’t scroll through its contents.
- Don’t open more than one drawer at a time.
Implementation Code
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
final appTitle = 'Drawer Demo';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: appTitle,
home: MyHomePage(title: appTitle),
theme: ThemeData(
primaryColor: Color(0xFF6200EE),
),
);
}
}
class MyHomePage extends StatefulWidget {
final String title;
MyHomePage({Key key, this.title}) : super(key: key);
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _selectedDestination = 0;
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
final textTheme = theme.textTheme;
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
drawer: Drawer(
child: ListView(
// Important: Remove any padding from the ListView.
padding: EdgeInsets.zero,
children: <Widget>[
Padding(
padding: const EdgeInsets.all(16.0),
child: Text(
'Header',
style: textTheme.headline6,
),
),
Divider(
height: 1,
thickness: 1,
),
ListTile(
leading: Icon(Icons.favorite),
title: Text('Item 1'),
selected: _selectedDestination == 0,
onTap: () => selectDestination(0),
),
ListTile(
leading: Icon(Icons.delete),
title: Text('Item 2'),
selected: _selectedDestination == 1,
onTap: () => selectDestination(1),
),
ListTile(
leading: Icon(Icons.label),
title: Text('Item 3'),
selected: _selectedDestination == 2,
onTap: () => selectDestination(2),
),
Divider(
height: 1,
thickness: 1,
),
Padding(
padding: const EdgeInsets.all(16.0),
child: Text(
'Label',
),
),
ListTile(
leading: Icon(Icons.bookmark),
title: Text('Item A'),
selected: _selectedDestination == 3,
onTap: () => selectDestination(3),
),
],
),
),
body: GridView.count(
crossAxisCount: 2,
crossAxisSpacing: 20,
mainAxisSpacing: 20,
padding: EdgeInsets.all(20),
childAspectRatio: 3 / 2,
children: [
Image.asset('assets/nav-drawer-1.jpg'),
Image.asset('assets/nav-drawer-2.jpg'),
Image.asset('assets/nav-drawer-3.jpg'),
Image.asset('assets/nav-drawer-4.jpg'),
],
),
);
}
void selectDestination(int index) {
setState(() {
_selectedDestination = index;
});
}
}
Conclusion
To conclude, remember that navigation drawers can utilize text labels without icons. Additionally, you must allow scrolling of the contents of a bottom drawer when it is at full height. If a navigation drawer takes up the entire vertical height of the page, a branding element or product name can be placed in the header space. To this end, if you found this useful, you can share it with your friends and family. Obviously, if you found it outdated, you can check out the official documentation here. Thank you for reading!