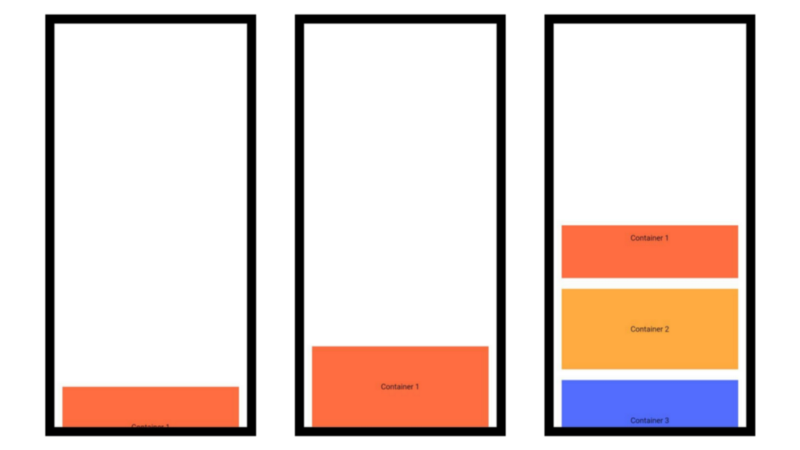
First, what is a DraggableScrollableSheet widget? Basically, it is a scrollable container that responds to dragging motions by resizing the scrollable until a limit is reached, then scrolls. This blog will discuss and teach how to use DraggableScrollableSheet in Flutter. You can also check out the documentation on how to use DraggableScrollableSheet in Flutter here. https://api.flutter.dev/flutter/widgets/DraggableScrollableSheet-class.html
DraggableScrollableSheet Arguments
const DraggableScrollableSheet({
Key key,
this.initialChildSize = 0.5,
this.minChildSize = 0.25,
this.maxChildSize = 1.0,
this.expand = true,
@required ScrollableWidgetBuilder this.builder,
})
There are three import arguments that you have to define in order to use the DraggableScrollable widget: the “initialChildSize”, “minChildSize”, and “maxChildSize”.
initialChildSize
The initialChildSize argument specifies how much of the DraggableScrollableSheet should be displayed on the screen at first. This is done so that users are aware that there is something they can interact with at the start of a sheet.
minChildSize
This parameter defines how much of a DraggableScrollableSheet’s child should be displayed when the user drags it down. Using this one, you can force the least amount of content to display even if the user has finished examining the contents or is attempting to swipe down the sheet.
maxChildSize
The maxChildSize property specifies how much of a widget’s height should be displayed when the widget is fully dragged. This allows users to explore other material within.
Let’s Code!
Suppose I want to write an app that looks like this:
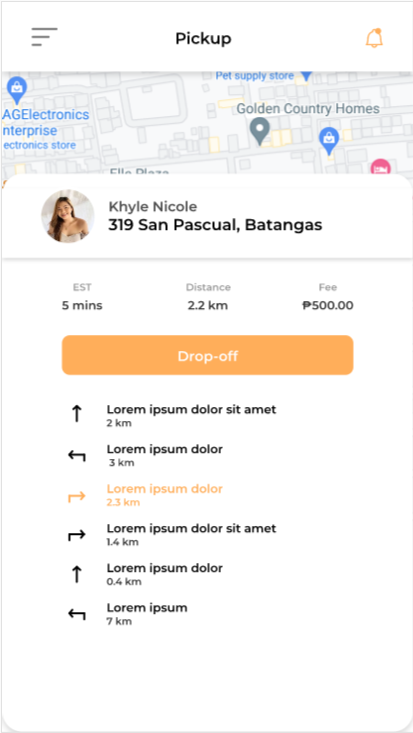
Accordingly, It is a draggable and scrollable column widget from the bottom section of the app. To achieve this, I need columns, rows, images, elevated buttons, text, icons, and finally the DraggableScrollableSheet widget. Check the code below on how to use DraggableScrollableSheet in Flutter.
import 'package:flutter/material.dart';
import 'package:nucleio_move/../directiontile.dart';
class PickUpScrollablePanel extends StatefulWidget {
String username = "";
String address = "";
String est = "";
String distance = "";
String fee = "";
PickUpScrollablePanel(
{Key? key,
required this.username,
required this.address,
required this.est,
required this.distance,
required this.fee})
: super(key: key);
@override
State<PickUpScrollablePanel> createState() => _PickUpScrollablePanelState();
}
class _PickUpScrollablePanelState extends State<PickUpScrollablePanel> {
@override
Widget build(BuildContext context) {
return DraggableScrollableSheet(
initialChildSize: 0.13,
minChildSize: 0.1,
maxChildSize: 0.8,
builder: (BuildContext context, ScrollController scrollController) {
return ListView(
controller: scrollController,
children: [
Container(
color: Colors.white,
child: Column(
children: [
Container(
alignment: Alignment.topCenter,
width: double.infinity,
height: 80,
decoration: BoxDecoration(
color: Colors.white,
boxShadow: [
BoxShadow(
color: Colors.grey.withOpacity(0.5),
spreadRadius: 5,
blurRadius: 7,
),
],
border: Border.all(
color: Colors.white,
),
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(
20,
),
topRight: Radius.circular(
20,
),
),
),
child: Padding(
padding: const EdgeInsets.fromLTRB(50, 10, 50, 10),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
CircleAvatar(
backgroundColor: Colors.white,
radius: 28,
child: CircleAvatar(
backgroundColor: Colors.blue,
radius: 25,
child: Image.asset(
'images/useravatar.png',
),
),
),
const SizedBox(
width: 10,
),
Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(
height: 10,
),
SizedBox(
width: 100,
child: Text(
widget.username,
style: const TextStyle(
color: Colors.grey,
fontSize: 15,
fontWeight: FontWeight.normal),
textAlign: TextAlign.start,
),
),
SizedBox(
width: 200,
child: Text(
widget.address,
style: const TextStyle(
color: Colors.black,
fontSize: 15,
fontWeight: FontWeight.bold),
textAlign: TextAlign.start,
),
),
],
)
],
),
],
),
),
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const SizedBox(
height: 20,
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Column(
children: [
const Text(
"EST",
style: TextStyle(
color: Colors.grey,
fontSize: 13,
fontWeight: FontWeight.normal),
textAlign: TextAlign.start,
),
Text(
widget.est,
style: const TextStyle(
color: Colors.black,
fontSize: 13,
fontWeight: FontWeight.bold),
textAlign: TextAlign.start,
)
],
),
Column(
children: [
const Text(
"Distance",
style: TextStyle(
color: Colors.grey,
fontSize: 13,
fontWeight: FontWeight.normal),
textAlign: TextAlign.start,
),
Text(
widget.distance,
style: const TextStyle(
color: Colors.black,
fontSize: 13,
fontWeight: FontWeight.bold),
textAlign: TextAlign.start,
)
],
),
Column(
children: [
const Text(
"Fee",
style: TextStyle(
color: Colors.grey,
fontSize: 13,
fontWeight: FontWeight.normal),
textAlign: TextAlign.start,
),
Text(
widget.fee,
style: const TextStyle(
color: Colors.black,
fontSize: 13,
fontWeight: FontWeight.bold),
textAlign: TextAlign.start,
),
],
),
],
),
const SizedBox(
height: 20,
),
SizedBox(
width: 250,
height: 35,
child: ElevatedButton(
onPressed: () {},
child: const Text(
"Drop-off",
style: TextStyle(
color: Colors.white,
fontSize: 20,
fontWeight: FontWeight.normal),
textAlign: TextAlign.center,
),
),
),
const SizedBox(
height: 20,
),
Column(
children: [
DirectionTile(
arrowdirection: Icon(Icons.arrow_upward),
directiontext: "Lorem ipsum dolor sit amet",
directiondistance: "2 KM"),
DirectionTile(
arrowdirection: Icon(Icons.arrow_left),
directiontext: "Lorem ipsum dolor",
directiondistance: "3 KM"),
DirectionTile(
arrowdirection: Icon(Icons.arrow_right),
directiontext: "Lorem ipsum dolor",
directiondistance: "2.3 KM"),
DirectionTile(
arrowdirection: Icon(Icons.arrow_right),
directiontext: "Lorem ipsum dolor sit amet",
directiondistance: "1.4 KM"),
DirectionTile(
arrowdirection: Icon(Icons.arrow_upward),
directiontext: "Lorem ipsum dolor",
directiondistance: "0.4 KM"),
DirectionTile(
arrowdirection: Icon(Icons.arrow_left),
directiontext: "Lorem ipsum",
directiondistance: "7 KM"),
],
),
],
),
],
),
),
],
);
},
);
}
}
Result
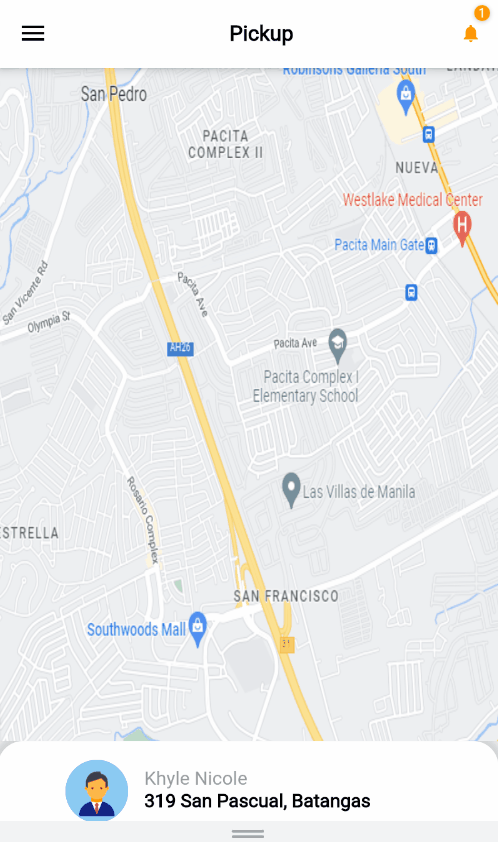
Conclusion
To conclude, I’m sure you’ve seen something like to this here and there, and believe it or not, it needs to be fulfilled as UX ambitions. This is how to use DraggableScrollable in Flutter.
In short , building similar things can be difficult on other platforms. Having said that, kudos to Flutter Folks for creating something as simple as its name suggests. Thank you for your time. Please share this post with your friends if you find it useful. Goodbye.