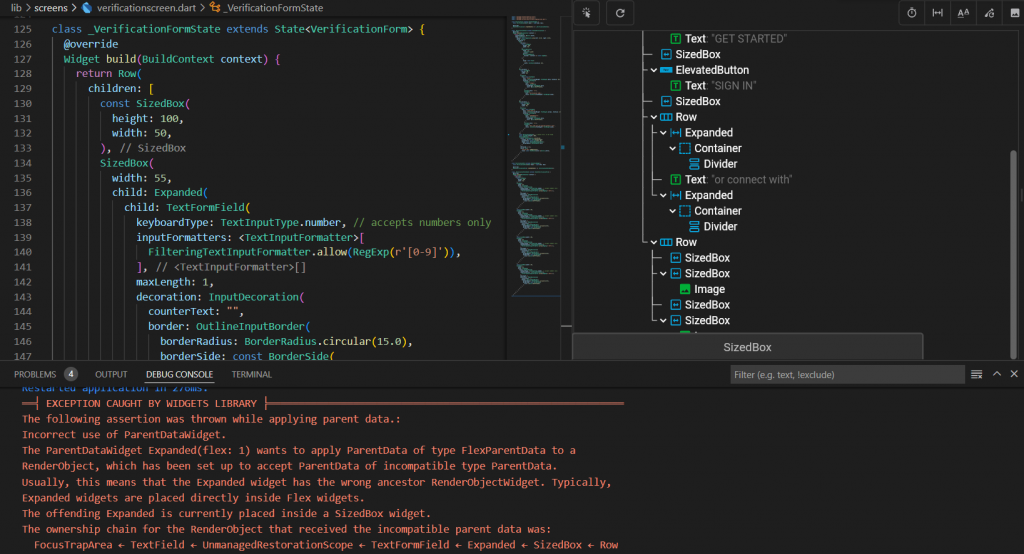
Yesterday I encounter this error and today I specifically found a way to literally fix it and I definitely am going to share it to you for those who also struggles with it in a subtle way.
The Error
The error says “exception caught by widgets library” or “Exception was thrown: Incorrect use of ParentDataWidget”.
The full error message was:
════════ Exception caught by widgets library ═══════════════════════════════════
The following assertion was thrown while applying parent data.:
Incorrect use of ParentDataWidget.
The ParentDataWidget Expanded(flex: 1) wants to apply ParentData of type FlexParentData to a RenderObject, which has been set up to accept ParentData of incompatible type ParentData.
Usually, this means that the Expanded widget has the wrong ancestor RenderObjectWidget. Typically, Expanded widgets are placed directly inside Flex widgets.
The offending Expanded is currently placed inside a SizedBox widget.
The ownership chain for the RenderObject that received the incompatible parent data was:
FocusTrapArea ← TextField ← UnmanagedRestorationScope ← TextFormField ← Expanded ← SizedBox ← Row ← VerificationForm ← Column ← Padding ← ⋯
When the exception was thrown, this was the stack
C:/b/s/w/ir/cache/builder/src/out/host_debug/dart-sdk/lib/_internal/js_dev_runtime/private/ddc_runtime/errors.dart 251:49 throw_
packages/flutter/src/widgets/framework.dart 5922:11 <fn>
packages/flutter/src/widgets/framework.dart 5938:14 [_updateParentData]
packages/flutter/src/widgets/framework.dart 5961:50 attachRenderObject
packages/flutter/src/widgets/framework.dart 5635:5 mount
packages/flutter/src/widgets/framework.dart 6283:11 mount
packages/flutter/src/widgets/framework.dart 3790:13 inflateWidget
packages/flutter/src/widgets/framework.dart 3540:18 updateChild
packages/flutter/src/widgets/framework.dart 4780:16 performRebuild
packages/flutter/src/widgets/framework.dart 4928:11 performRebuild
packages/flutter/src/widgets/framework.dart 4477:5 rebuild
packages/flutter/src/widgets/framework.dart 4735:5 [_firstBuild]
packages/flutter/src/widgets/framework.dart 4919:11 [_firstBuild]
packages/flutter/src/widgets/framework.dart 4729:5 mount
packages/flutter/src/widgets/framework.dart 3790:13 inflateWidget
packages/flutter/src/widgets/framework.dart 3540:18 updateChild
packages/flutter/src/widgets/framework.dart 4780:16 performRebuild
packages/flutter/src/widgets/framework.dart 4477:5 rebuild
packages/flutter/src/widgets/framework.dart 4735:5 [_firstBuild]
packages/flutter/src/widgets/framework.dart 4477:5 rebuild
packages/flutter/src/widgets/framework.dart 5108:5 update
packages/flutter/src/widgets/framework.dart 3501:14 updateChild
packages/flutter/src/widgets/framework.dart 4780:16 performRebuild
packages/flutter/src/widgets/framework.dart 4928:11 performRebuild
packages/flutter/src/widgets/framework.dart 4477:5 rebuild
packages/flutter/src/widgets/framework.dart 2659:18 buildScope
packages/flutter/src/widgets/binding.dart 882:9 drawFrame
packages/flutter/src/rendering/binding.dart 363:5 [_handlePersistentFrameCallback]
packages/flutter/src/scheduler/binding.dart 1144:15 [_invokeFrameCallback]
packages/flutter/src/scheduler/binding.dart 1081:9 handleDrawFrame
packages/flutter/src/scheduler/binding.dart 995:5 [_handleDrawFrame]
C:/b/s/w/ir/cache/builder/src/out/host_debug/flutter_web_sdk/lib/_engine/engine/platform_dispatcher.dart 1011:13 invoke
C:/b/s/w/ir/cache/builder/src/out/host_debug/flutter_web_sdk/lib/_engine/engine/platform_dispatcher.dart 159:5 invokeOnDrawFrame
C:/b/s/w/ir/cache/builder/src/out/host_debug/flutter_web_sdk/lib/_engine/engine/initialization.dart 128:45 <fn>
Most of it for all intents and purposes was gibberish and encountering this error at first for the most part is definitely frustrating because the problem isn”t exactly very clear to understand in a subtle way.
Source Code
To fix this error we must clearly understand the cause of it and following this source code will help us retrace back our steps on where we caused this error.
Row(
children: [
const SizedBox(
height: 100,
width: 50,
),
SizedBox(
width: 55,
child: Expanded(
child: TextFormField(
keyboardType: TextInputType.number, // accepts numbers only
inputFormatters: <TextInputFormatter>[
FilteringTextInputFormatter.allow(RegExp(r'[0-9]')),
],
maxLength: 1,
decoration: InputDecoration(
counterText: "",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(15.0),
borderSide: const BorderSide(
color: Color(0xFFffa242),
),
),
),
),
),
),
],
);
The Cause
This error happens when child widgets like Flexible(), Expanded(), Positioned() and TableCell() widget has no matching parent widget. If we relate to the source code this is definitely the cause of the error.
Solution
In my case, I fixed it by removing the SizedBox parent widget of my Expanded widget that wraps my TextFormField widget to determine its width, but I also attempted resizing it by simply placing another SizedBox after the TextFormField.
Row(
children: [
const SizedBox(
height: 100,
width: 50,
),
Expanded(
child: TextFormField(
keyboardType: TextInputType.number, // accepts numbers only
inputFormatters: <TextInputFormatter>[
FilteringTextInputFormatter.allow(RegExp(r'[0-9]')),
],
maxLength: 1,
decoration: InputDecoration(
counterText: "",
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(15.0),
borderSide: const BorderSide(
color: Color(0xFFffa242),
),
),
),
),
),
const SizedBox(
height: 100,
width: 50,
),
],
);
Conclusion
So don’t forget that using the SizedBox and Expanded widget comes with a cost that the widget must follow it’s matching parent.
Here are the sources of my research from where I am able to solve it.
https://www.fluttercampus.com/guide/229/incorrect-use-of-parentdatawidget-error/
https://www.kindacode.com/article/flutter-textfield-width-height-padding/
Thanks for reading, Until next time!
Thanks for this solution really helped me fix my issue