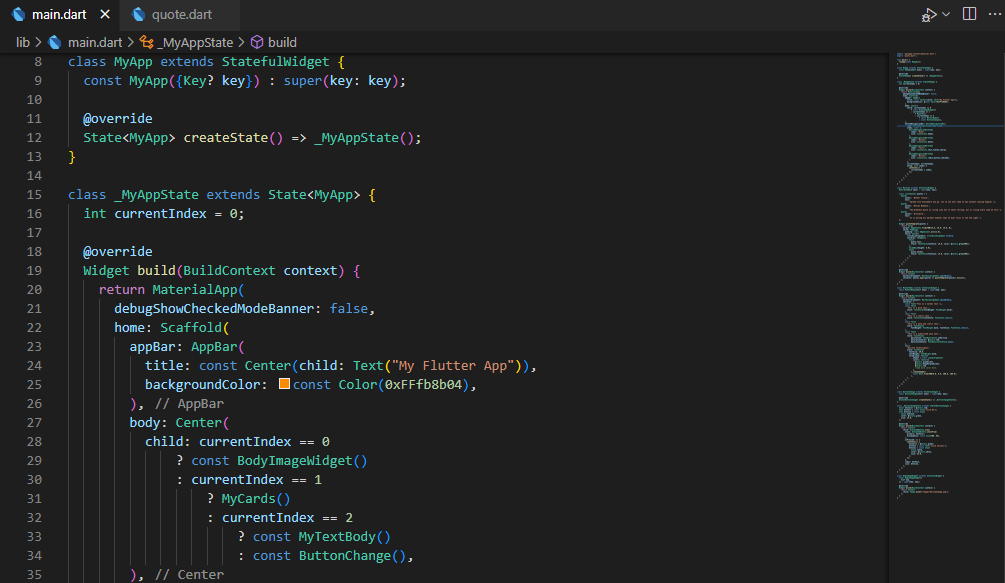
Today in this blog I am going to combine all the screens I’ve made in the recent blog post, a compilation of work app that we can navigate through the use of BottomNavigationBar widget.
BottomNavigationBarItem
From my recent blog I am going to change the BottomNavigationBarItem to this code:
BottomNavigationBarItem(
label: "Home",
icon: Icon(Icons.home),
),
BottomNavigationBarItem(
label: "Quotes",
icon: Icon(Icons.book),
),
BottomNavigationBarItem(
label: "Texts",
icon: Icon(Icons.text_fields_sharp),
),
BottomNavigationBarItem(
label: "Button",
icon: Icon(Icons.radio_button_checked),
)
Now the labels and icons are done. You’ll encounter a common bug in this widget. Fret not, you can fix it using this code to set your BottomNavigationBarItem type to “fixed’.
type: BottomNavigationBarType.fixed,
Body and Ternary Operator
On your “body” argument of your Flutter app, you need to write a ternary operator that switches screens or widget to show on the body portion of the app to do this I made this code:
body: Center(
child: currentIndex == 0
? const BodyImageWidget()
: currentIndex == 1
? MyCards()
: currentIndex == 2
? const MyTextBody()
: const ButtonChange(),
),
This will switch your view on the body by selecting on the BottomNavigationBar widget.
Result
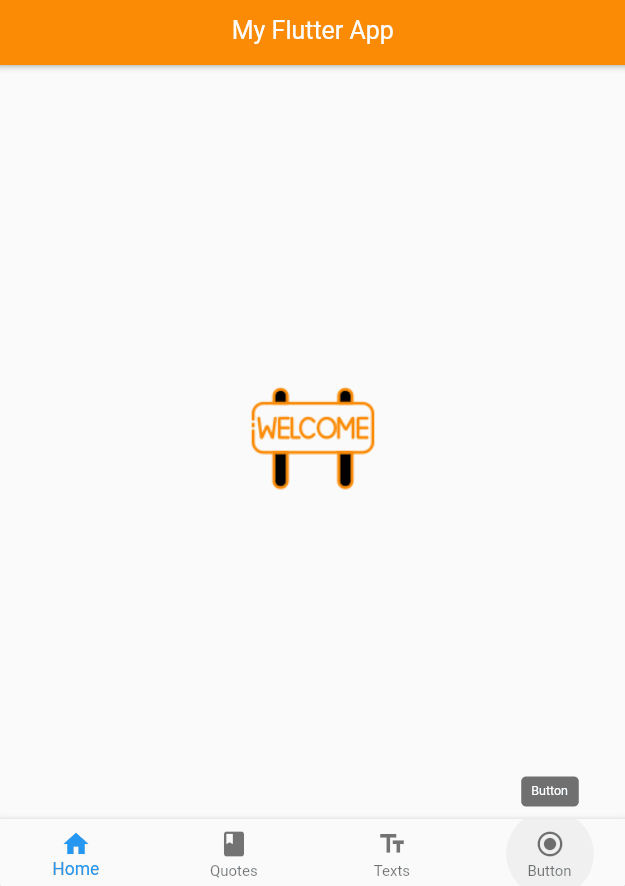
Nicely done! As you can see we can navigate through our screen using the BottomNavigationBar widget.
Source Code:
Here is the complete source code in case you need it.
import 'package:flutter/material.dart';
import 'quote.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({Key? key}) : super(key: key);
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
int currentIndex = 0;
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
appBar: AppBar(
title: const Center(child: Text("My Flutter App")),
backgroundColor: const Color(0xFFfb8b04),
),
body: Center(
child: currentIndex == 0
? const BodyImageWidget()
: currentIndex == 1
? MyCards()
: currentIndex == 2
? const MyTextBody()
: const ButtonChange(),
),
bottomNavigationBar: BottomNavigationBar(
type: BottomNavigationBarType.fixed,
items: const [
BottomNavigationBarItem(
label: "Home",
icon: Icon(Icons.home),
),
BottomNavigationBarItem(
label: "Quotes",
icon: Icon(Icons.book),
),
BottomNavigationBarItem(
label: "Texts",
icon: Icon(Icons.text_fields_sharp),
),
BottomNavigationBarItem(
label: "Button",
icon: Icon(Icons.radio_button_checked),
)
],
currentIndex: currentIndex,
onTap: (int index) {
setState(() {
currentIndex = index;
});
},
),
),
);
}
}
class MyCards extends StatelessWidget {
MyCards({Key? key}) : super(key: key);
final List<Quote> quotes = [
Quote(
author: 'Mother Teresa',
text:
'Spread love everywhere you go. Let no one ever come to you without leaving happier.'),
Quote(
author: 'Nelson Mandela',
text:
'The greatest glory in living lies not in never falling, but in rising every time we fall.'),
Quote(
author: 'Aristotle',
text:
'It is during our darkest moments that we must focus to see the light.')
];
Widget quoteTemplate(quote) {
return Card(
margin: EdgeInsets.fromLTRB(16.0, 16.0, 16.0, 0),
child: Padding(
padding: const EdgeInsets.all(12.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Text(
quote.text,
style: TextStyle(fontSize: 18.0, color: Colors.grey[600]),
),
SizedBox(height: 6.0),
Text(
quote.author,
style: TextStyle(fontSize: 14.0, color: Colors.grey[800]),
),
],
),
),
);
}
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: quotes.map((quote) => quoteTemplate(quote)).toList(),
);
}
}
class MyTextBody extends StatelessWidget {
const MyTextBody({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
const Text('This is a normal text.'),
const Text(
'This is a bold text.',
style: TextStyle(fontWeight: FontWeight.bold),
),
const Text(
'This is a italic text.',
style: TextStyle(fontStyle: FontStyle.italic),
),
const Text(
'This is a bold and italic text.',
style: TextStyle(
fontWeight: FontWeight.bold, fontStyle: FontStyle.italic),
),
const Text(
'This is a underlined wavy text.',
style: TextStyle(
decoration: TextDecoration.underline,
decorationColor: Colors.red,
decorationStyle: TextDecorationStyle.wavy),
),
Text(
"Nucleio Technologies",
style: TextStyle(
fontSize: 30.0,
fontWeight: FontWeight.bold,
foreground: Paint()
..shader = const LinearGradient(
colors: <Color>[
Colors.pinkAccent,
Colors.deepPurpleAccent,
Colors.red
//add more color here.
],
).createShader(
const Rect.fromLTWH(0.0, 0.0, 200.0, 100.0),
),
),
),
],
);
}
}
class ButtonChange extends StatefulWidget {
const ButtonChange({Key? key}) : super(key: key);
@override
State<ButtonChange> createState() => _ButtonChangeState();
}
class _ButtonChangeState extends State<ButtonChange> {
Color btnColor = Colors.red;
Text btnText = const Text('Click Me');
Icon btnIcon = const Icon(
Icons.android,
color: Colors.green,
size: 24.0,
);
@override
Widget build(BuildContext context) {
return Center(
child: ElevatedButton.icon(
style: ElevatedButton.styleFrom(
primary: btnColor,
minimumSize: const Size(200, 50),
),
onPressed: () {
setState(() {
btnColor = Colors.green;
btnText = const Text('Click Success');
btnIcon = const Icon(
Icons.check,
color: Colors.white,
size: 24.0,
);
});
},
label: btnText,
icon: btnIcon,
),
);
}
}
class BodyImageWidget extends StatelessWidget {
const BodyImageWidget({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Center(
child: Image.asset("images/WelcomeImage.png"),
);
}
}
//quote.dart
class Quote {
String? text; // errors need ?
String? author;
Quote({this.text, this.author});
}
Conclusion
I’ve made a compilation of my app in this blog post using BottomNavigationBar to switch different view on the body structure of the Flutter app and using the ternary operator to switch based on the current index it was selected.
Keep following me on my Flutter journey, Until next time, Farewell!