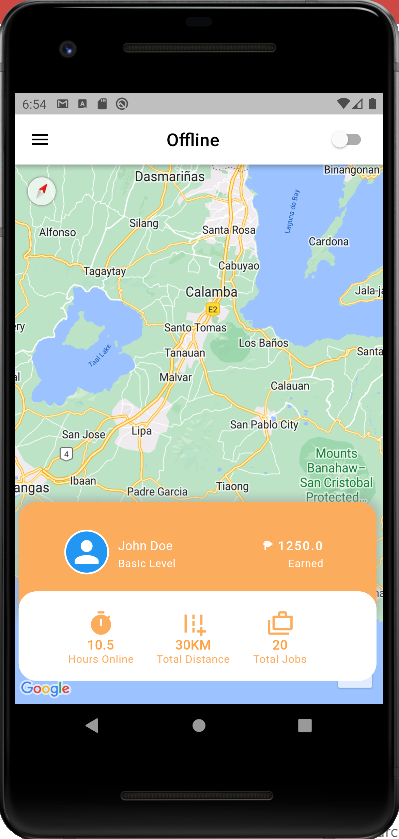
Today we’re going to add Google Maps to our Flutter app, specifically for Android users. The Google Maps API isn’t exactly free, but this information is still relevant as you could still apply it to your Flutter app. It is easy if you know where to get resources and updated information about this implementation of this API. As a result, the question of how to add Google Maps to Flutter arises. Let’s get started!
Google Maps Package
First, we must add the dependencies into our Flutter project. To do that, execute the code below inside your project directory.
flutter pub add google_maps_flutter
Additionally, after you add it, you can now import it into your project like the code below.
import 'package:google_maps_flutter/google_maps_flutter.dart';
AndroidManifest File Configuration
Next, we must edit our AndroidManifest file and add your Google Maps API key just like in the code below. It should be inside the application just after the activity tag.
<meta-data android:name="com.google.android.geo.API_KEY"
android:value="YOUR-KEY-HERE"/>
Add Google Maps In Flutter
Now that all the packages, imports, and configurations are done, we will use the Google Maps widget, then put it into our project below. Bear in mind that it needs a target parameter and an initial map parameter. Check the code below.
// code...
late GoogleMapController mapController;
final LatLng _center = const LatLng(45.521563, -122.677433);
void _onMapCreated(GoogleMapController controller) {
mapController = controller;
}
// codes...
// body: widget(_center, _onMapCreated)...
// Widget widget(map, target)...
// more codes...
Container(
width: double.infinity,
height: double.infinity,
child: Stack(
children: [
GoogleMap( // Google Maps code here
onMapCreated: map, // paremeter used
initialCameraPosition: CameraPosition(
target: target, // parameter used
zoom: 11.0,
),
),
Positioned(
left: constraints.maxWidth * 0.01,
bottom: (constraints.maxHeight * 0.01) - 30,
child: UserFloatingPanel(
username: "John Doe",
userlevel: "Basic Level",
userearnings: 1250.00,
subtext: "Earned"),
),
],
),
);
Conclusion
The API isn’t free, but this information is still relevant as you could still apply it to your Flutter app. It is easy if you know where to get resources and updated information about this implementation of this API. The code above shows how to add Google Maps to Flutter. If you want to style it, you can check out this blog here on how to do so. If you find this blog outdated in the future, you can always check out the official documentation. Thank you for reading. Please share it if you found it useful.