Version 0.5.16 of the google_maps_flutter plugin has arrived and it includes the ability to add a custom style to your map! This article will describe an easy way to style your Google Map in Flutter.
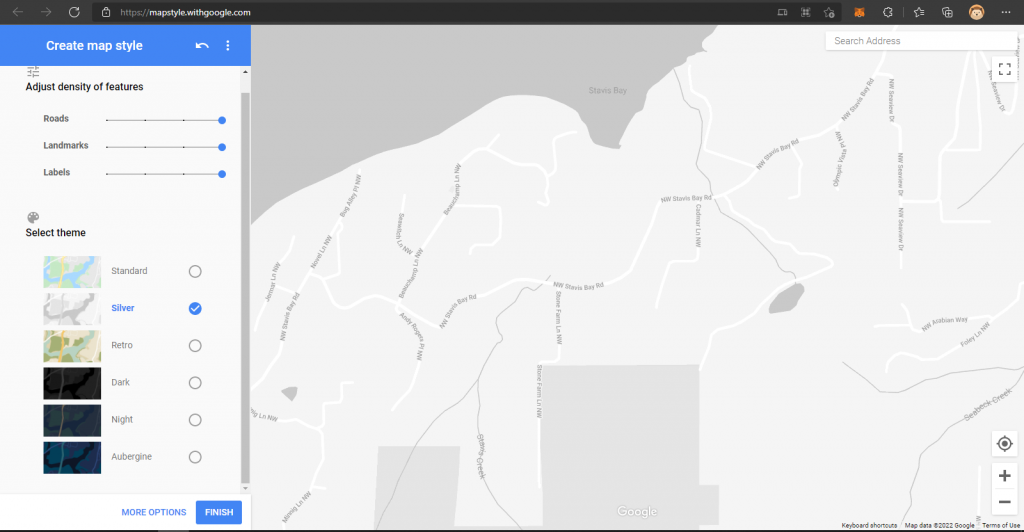
The new plugin version adds the setMapStyle method to the GoogleMapController class. This method takes the String representation of your custom style as input. To create this style in JSON format, Google created a handy tool: https://mapstyle.withgoogle.com/. You can use any of the pre-made themes, or create your own style in detail. After you’ve created your style, click finish and you can copy the resulting JSON. Following JSON represents a simple style that sets the water color to grey and roads to white:
[
{
"elementType": "geometry",
"stylers": [
{
"color": "#f5f5f5"
}
]
},
{
"featureType": "road",
"elementType": "geometry",
"stylers": [
{
"color": "#ffffff"
}
]
},
{
"featureType": "water",
"elementType": "geometry",
"stylers": [
{
"color": "#c9c9c9"
}
]
},
{
"featureType": "water",
"elementType": "labels.text.fill",
"stylers": [
{
"color": "#9e9e9e"
}
]
}
]
Now you can try to place this JSON in quotation marks and feed it directly to the setMapStyle method of your GoogleMapController. I will lay out an alternative way that is a little bit more handy in my opinion, by treating the style as an asset.
Copy the JSON and paste it into your editor of choice, such as Notepad or VS Code and save the file in txt format. Add this file to the assets of your Flutter project, by placing it in your assets folder and specifying it in your pubspec.yaml file. If your folder is called assets:
flutter:
assets:
- assets/map_style.txt
When you want to change your style from now on, you can simply edit it from this file. Now we’re ready to read this file as a String.
In the State class where your GoogleMap widget resides, add the following import statement:
import 'package:flutter/services.dart' show rootBundle;
And add a field that will represent your style in String format:
String _mapStyle;
We can now load the txt file as a String and feed it to the _mapStyle field. We do this from within the initState method of the State class:
@override
void initState() {
super.initState();
rootBundle.loadString('assets/map_style.txt').then((string) {
_mapStyle = string;
});
}
Now when you initialize the GoogleMap widget and its corresponding GoogleMapController, set the style as follows:
GoogleMapController mapController;
GoogleMap(
onMapCreated: (GoogleMapController controller) {
mapController = controller;
mapController.setMapStyle(_mapStyle);
}
);
And you’re done! The map will now load your custom style and you can simply edit the style from within map_style.txt when needed.
Reference:
https://mapstyle.withgoogle.com/
https://medium.com/@matthiasschuyten/google-maps-styling-in-flutter-5c4101806e83