Tkinter is a Python library that allows you to create graphical user interfaces (GUIs) for desktop applications. Here are the basic steps to create a simple Tkinter GUI;
Import the Tkinter library
import tkinter as tk
Create the main window
root = tk.Tk()
Set the window title
root.title("My Tkinter Window")
Create a label widget
label = tk.Label(root, text="Hello, World!")
Add the label widget to the window
label.pack()
Start the event loop
root.mainloop()
This will create a simple window with a label that says “Hello, World!”.
You can customize the window and widgets using various Tkinter options and methods. For example, you can set the window size and background color;
root.geometry("400x300")
root.configure(bg="white")
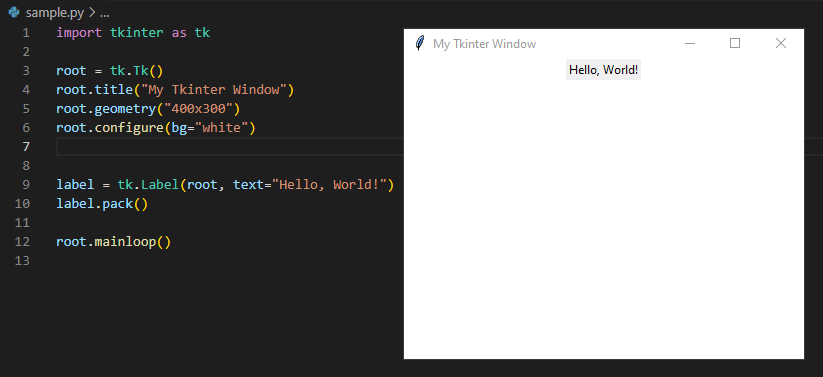
You can also add buttons, text boxes, images, and other widgets to the window, and define event handlers for user interactions.
Tkinter provides many built-in widgets and layout managers, which you can use to create complex GUIs with ease. For more information and examples, check out the official Tkinter documentation.
Reference