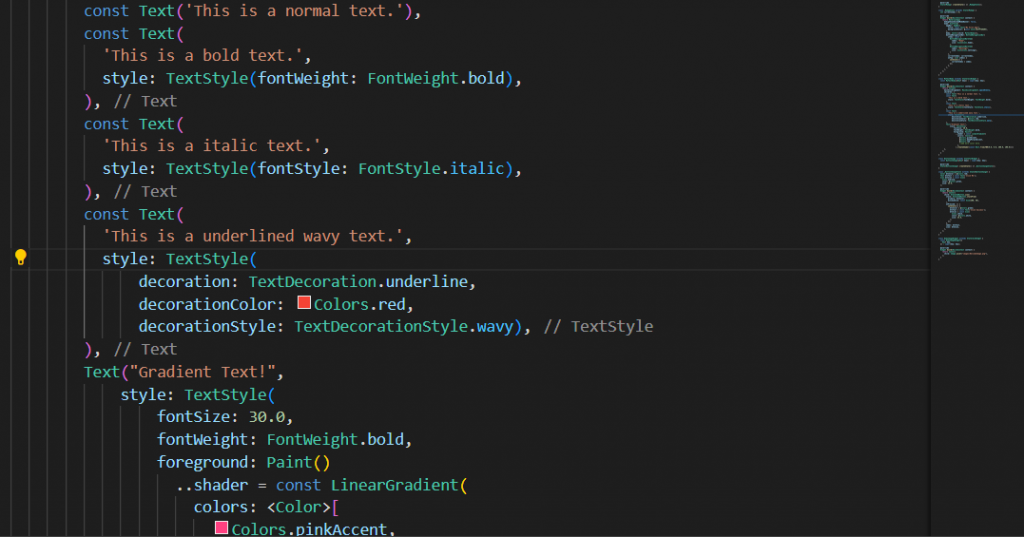
Today we’re focusing on TextStyles. Every app has a text but everyone should know how to style them. That is why I am going to share how to style them. Let’s go!
FontWeight and FontStyle
You probably already know how to make normal Text like this:
const Text('This is a normal text.')
This is how to make it bold:
const Text(
'This is a bold text.',
style: TextStyle(fontWeight: FontWeight.bold),
)
And this is how to make it italic:
const Text(
'This is a italic text.',
style: TextStyle(fontStyle: FontStyle.italic),
)
But you can also combine them:
const Text(
'This is a bold and italic text.',
style: TextStyle(fontWeight: FontWeight.bold, fontStyle: FontStyle.italic),
)
You can also apply decoration in them like this:
const Text(
'This is a underlined wavy text.',
style: TextStyle(
decoration: TextDecoration.underline,
decorationColor: Colors.red,
decorationStyle: TextDecorationStyle.wavy),
)
Advanced Style
This one is a little advance I am using the Paint() class for the foreground argument and LinearGradient in shader arguments and a list of colors. Here’s the code:
Text(
"Nucleio Technologies",
style: TextStyle(
fontSize: 30.0,
fontWeight: FontWeight.bold,
foreground: Paint()
..shader = const LinearGradient(
colors: <Color>[
Colors.pinkAccent,
Colors.deepPurpleAccent,
Colors.red
//add more color here.
],
).createShader(
const Rect.fromLTWH(0.0, 0.0, 200.0, 100.0),
),
),
)
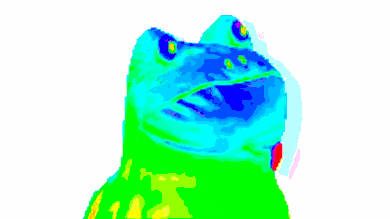
Nicely done! Now if you follow through that you got yourself a fancy gradient text!
Here’s the full application look like:
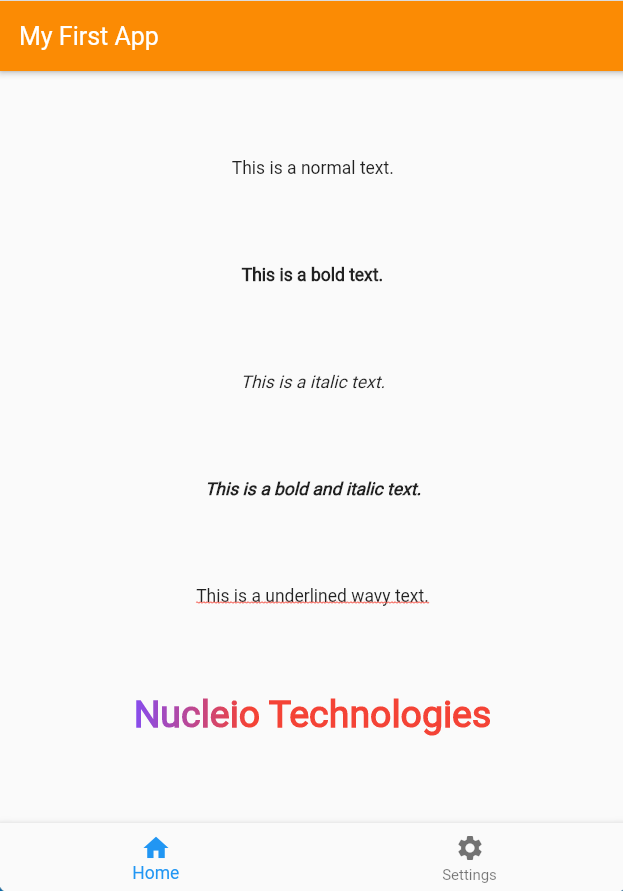
Conclusion
I’ve covered the basics of styling the Text widget in Flutter and I also added how to apply a linear gradient on the text.
You could also read the docs here incase there’s any changes in the future:
https://api.flutter.dev/flutter/painting/TextStyle-class.html
That’s all for today, Keep following me on my Flutter journey.
Until next time, Farewell!