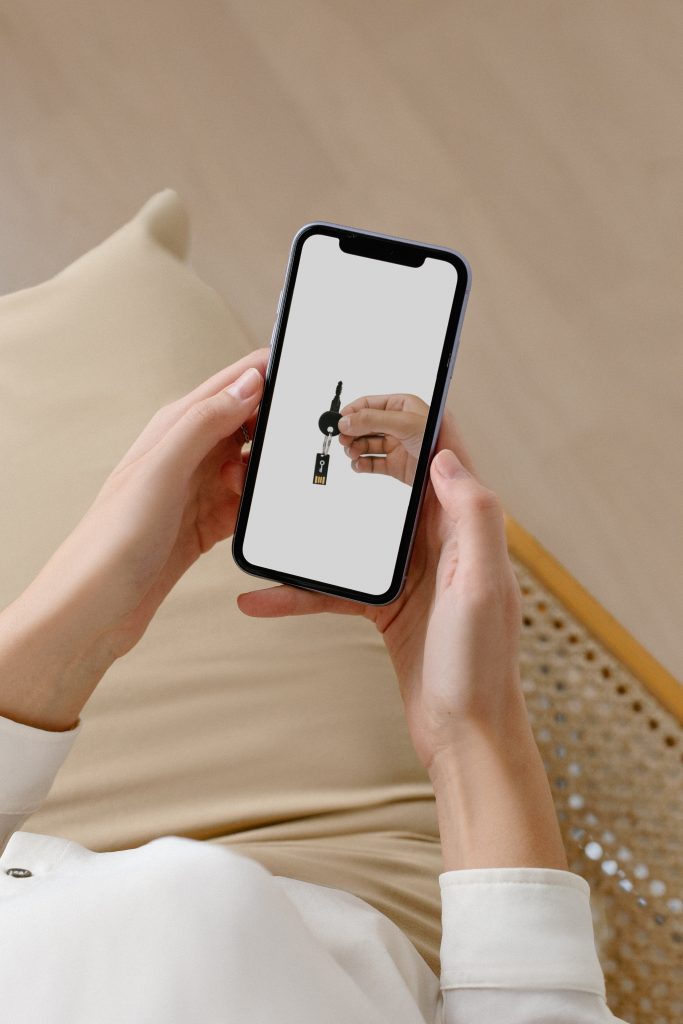
You might already know how to read and write data in Flutter, but every now and then we need to store it in a key-value pair. That is why today I’m going to discuss how to store key-value pairs in Flutter. Let’s get started!
The shared_preferences
plugin can be used to save a small collection of key-value pairs. Normally, you’d have to create native platform integrations for both iOS and Android to save data. Fortunately, the shared_preferences
plugin allows you to save key-value data to disk. The shared_preferences
plugin provides a persistent store for simple data by wrapping NSUserDefaults
on iOS and SharedPreferences
on Android.
Dependency
Before you begin, include the shared preferences plugin in the pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
shared_preferences: "<newest version>"
You can also run this command in your project directory:
flutter pub add shared_preferences
Store Key-Value Pairs
Use the setter methods given by the SharedPreferences
class to persist values. Setter methods exist for a variety of primitive types, including setInt
, setBool
, and setString
.
Setter methods perform two functions. The first is to synchronously update the key-value pair in memory. The data should then be saved to disk.
// obtain shared preferences
final prefs = await SharedPreferences.getInstance();
// set value
await prefs.setInt('counter', counter);
Read Key-Value Pairs
To read data, use the SharedPreferences
class’s appropriate getter method. Each setter has a corresponding getter. You can, for example, use the getInt
, getBool
, and getString
functions.
final prefs = await SharedPreferences.getInstance();
// Try reading data from the counter key. If it doesn't exist, return 0.
final counter = prefs.getInt('counter') ?? 0;
Remove Key-Value Pairs In Flutter
Simply use the remove() function to delete data.
final prefs = await SharedPreferences.getInstance();
await prefs.remove('counter');
Conclusion and Precautions
To conclude, we already know how to store, read, and remove key-value pairs in Flutter. Although key-value storage is easy and convenient to use, it has limitations, which are that it can only use primitive types and it’s not designed to store a lot of data. You can also test it by going to the official documentation and testing section. That would be all. Thank you for reading. If you found this useful, please share it.