Flutter uses the TextField and TextFormField widget to allow users to input and submit data. When working with TextFields, you may have had to use multiple of them in one Row widget. However, when not done in the right way, this might produce the InputDecorator error.
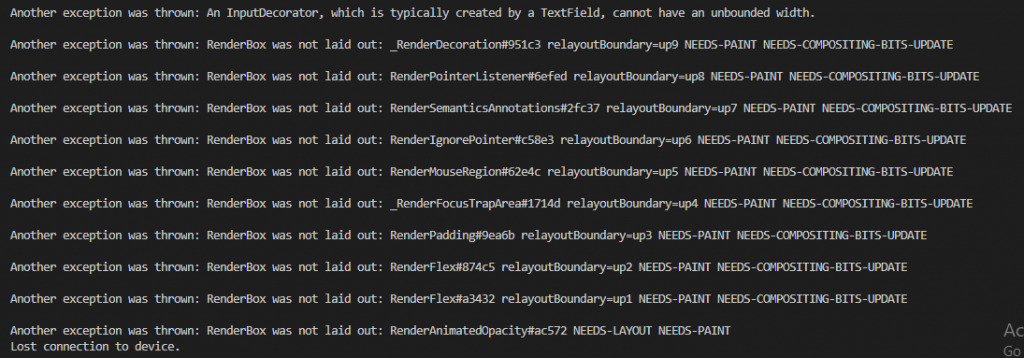
As seen in the image above, it might also produce more errors depending on how your code was made. This error appears when you use a TextField inside a Row due to TextField taking all spaces in a horizontal direction. Due to Row not adding a constraint on its children’s width, it is difficult for a Flutter program to estimate the size of a TextField. Fortunately, there are two easy ways to fix this.
Using SizedBox
One way to fix this is to use SizedBox and adding the width parameter.
Code Example:
Row(
children: const <Widget>[
SizedBox (
width: 300,
child: TextField(
decoration: InputDecoration(helperText: “Enter your name: “),
),
),
],
)
The SizedBox widget makes it so that the TextField is displayed in a limited width instead of having infinite size.
Using Expanded
Another way is to use Expanded to make the TextField widget occupy all the spaces that are available in the horizontal direction.
Code Example:
Row(
children: const <Widget>[
Expanded(
child: TextField(
decoration: InputDecoration (helperText: “Enter your name:),
),
),
]
)
Conclusion
By learning how to fix this error, we can now freely use as many TextFields as we want without producing any error. The SizedBox and Expanded widgets are very important when coding with Flutter. By determining the solution that is best fit for your code, you will know how to fix the issues without producing any more errors.