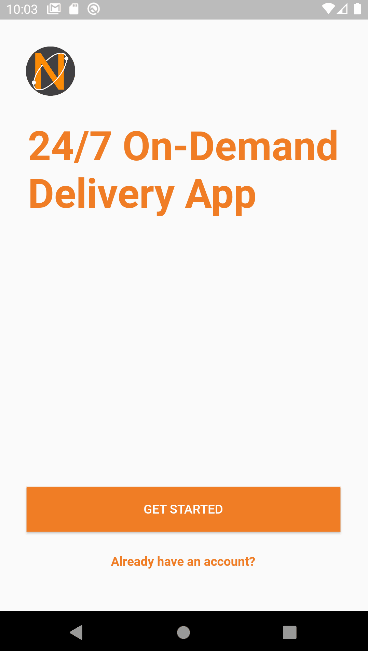
Every app nowadays has a transition for changing the screens. That is why we’re going to tackle how to add transition in flutter so we can also apply it to our flutter projects. In fact, this blog will tackle two simple transitions, which are the scale transistion and the slide transistion, on all sides. Let’s get started!
Custom Page Route & Scale Transistion
Accordingly, to add transition in Flutter when changing screens, you will need to create a new class which is a new dart file, extend that class into the PageRouteBuilder
class, and make sure it can receive a child widget which is the screen you want it to jump through between the transition animation.
Additionally, you must override the buildTransistion
method of the PageRouteBuilder
class and apply your own animations there, like in the code below. The example has a scale transition animation.
import 'package:flutter/material.dart';
class ScaleTransistionPageRoute extends PageRouteBuilder {
final Widget child;
ScaleTransistionPageRoute({
required this.child,
}) : super(
transitionDuration: const Duration(milliseconds: 500),
reverseTransitionDuration: const Duration(milliseconds: 500),
pageBuilder: (context, animation, secondaryAnimation) => child);
@override
Widget buildTransitions(BuildContext context, Animation<double> animation,
Animation<double> secondaryAnimation, Widget child) =>
ScaleTransition(scale: animation, child: child,);
}
To an extent, if you checked the code above, you can set the transition duration and its reverse duration using the Duration
widget, which can be any duration like seconds, milliseconds, etc. You name it.
Slide Transition In Flutter
On the other hand, the slide transition has a little more complexity compared to the scale transition. The scale transition needs a direction where it can begin on its slide transition, which you can define using the Offset widget. Thankfully, you can make this more readable using the AxisDirection widget, but you may need to create a function where when you pass such a widget it will return its offset location, which can be done using switch. Additionally, you may check the code below where all of it is ready for you to use. You’re welcome.
import 'package:flutter/material.dart';
class SlideTransistionPageRoute extends PageRouteBuilder {
final Widget child;
final AxisDirection direction;
SlideTransistionPageRoute({
required this.child,
this.direction = AxisDirection.right,
}) : super(
transitionDuration: const Duration(milliseconds: 500),
reverseTransitionDuration: const Duration(milliseconds: 500),
pageBuilder: (context, animation, secondaryAnimation) => child);
@override
Widget buildTransitions(BuildContext context, Animation<double> animation,
Animation<double> secondaryAnimation, Widget child) =>
SlideTransition(
position: Tween<Offset>(begin: getBeginOffset(), end: Offset.zero)
.animate(animation),
child: child,
);
Offset getBeginOffset() {
switch (direction) {
case AxisDirection.up:
return const Offset(0, 1);
case AxisDirection.down:
return const Offset(0, -1);
case AxisDirection.right:
return const Offset(-1, 0);
case AxisDirection.left:
return const Offset(1, 0);
}
}
}
Adding The Transition In Flutter
Finally, to add the transitions we made, we can simply replace our MaterialPageRoute widget into the custom page route we made. Simply refer to the code below.
// Normal Material Transistion
onPressed: () {
Navigator.of(context).push(
MaterialPageRoute(
builder: (BuildContext context) => const SignUp()
),
);
},
// Slide Transistion
onPressed: () {
Navigator.of(context).push(
SlideTransistionPageRoute(
child: SignUp(),
direction: AxisDirection.up, // set directions here
),
);
},
// Scale Transistion
onPressed: () {
Navigator.of(context).push(
ScaleTransistionPageRoute(
child: SignUp(),
),
);
},
Conclusion
To conclude, this blog tackled how to add transitions in flutter so we can also apply them to our flutter projects. We made two simple transitions, which are the scale transition and the slide transition. But before any of it, we created a custom page route class first so we could apply the transitions. The code given on this blog was flexible and ready to be used by the readers. Thank you for reading. If you find this useful, please share it, and you may also consider following me on my weekday blog posting here.
Referenced From: https://github.com/JohannesMilke/route_transition_example