We will be able to generate custom icons through FlutterIcon.com. It allows us to either:
- Upload a SVG that gets converted into an icon
- Choose from a huge selection of icons from a different set of icon packages
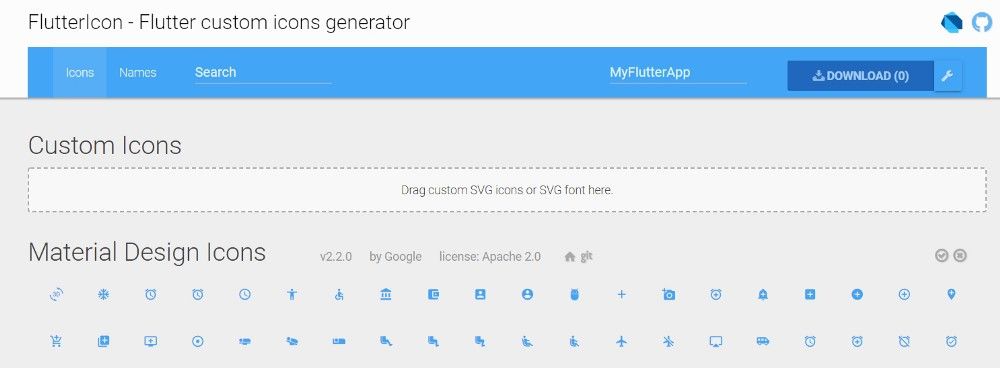
☝️ There is a package called FlutterIcon that has all of the icons shown, but due to it’s heavy size, I recommended only choosing the icons that you need and not using it.
Let’s demonstrate how to import custom icons into your application using this website.
Imagine we have the following form in our application:

You can see that we used icons for each TextFormField. Below is the code for the first TextFormField:
TextFormField(
controller: pillNameTextEditingController,
decoration: const InputDecoration(
border: OutlineInputBorder(),
hintText: 'What is the pill\'s name?',
prefixIcon: Icon(Icons.title)
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please enter a pill name';
}
return null;
}
)
How about we change the first TextFormField’s icon into something more relevant?
On FlutterIcon.com:
- Choose the icons that you want to use/upload a SVG file
- Give a meaningful name to your icon class (We’ll call our class CustomIcons)
- Press Download

In the .zip folder that you downloaded, there are several files:
- A fonts folder with a TTF file with the name of the class you chose
- A config.json file that’s used to remember what icons you chose
- A dart class with the name of the class you chose

Inside your project, import the .ttf file into a folder called fonts under the root directory. It should look like this:
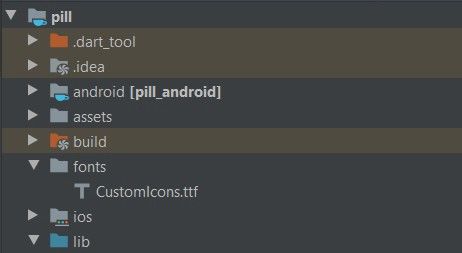
Place the .dart class inside your lib folder. If you take a look inside the dart file, you will see something similar (you might see more IconData objects if you chose more than one icon to download):
import 'package:flutter/widgets.dart';
class CustomIcons {
CustomIcons._();
static const _kFontFam = 'CustomIcons';
static const String? _kFontPkg = null;
static const IconData pill = IconData(0xea60, fontFamily: _kFontFam, fontPackage: _kFontPkg);
}
Add the following to your pubspec.yaml file:
fonts:
- family: CustomIcons
fonts:
- asset: fonts/CustomIcons.ttf
Run flutter pub get
in the terminal or click Pub get inside the IDE.
Go to the place where you want to use your custom icons and use it like this:
n.dart
TextFormField(
controller: pillNameTextEditingController,
decoration: const InputDecoration(
border: OutlineInputBorder(),
hintText: 'What is the pill\'s name?',
prefixIcon: Icon(CustomIcons.pill)
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please enter a pill name';
}
return null;
}
)
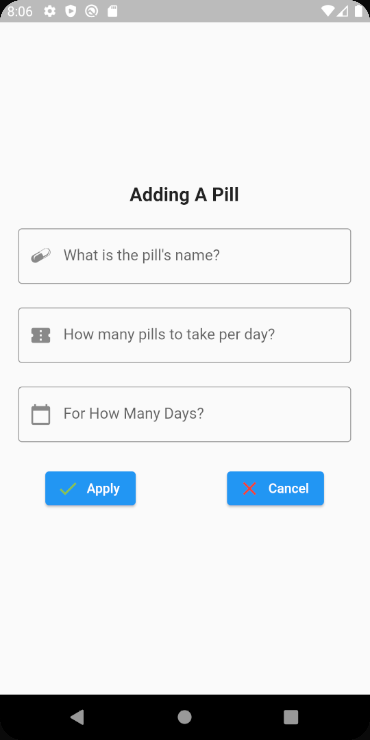
Reference
https://www.freecamp.org/news/content/images/2022/01/1_cUVrtz2mfcum4hsK44kDNA.jpeg
https://www.frsadp.org/news/content/images/2022/01/1_G7MmSKn3xVeJJcLkUO0goA.png