Flutter is renowned for its fast development and high-performance applications. However, as apps grow in complexity, maintaining optimal performance becomes crucial. This guide explores best practices and tips for enhancing Flutter app performance.
Flutter’s performance is critical for delivering a smooth user experience across different devices and platforms. By following these best practices and tips, you can optimize your Flutter app for better performance and responsiveness.
1. Minimize Widget Rebuilds
Flutter’s declarative UI model rebuilds widgets when state changes occur. However, excessive widget rebuilding can impact performance. To minimize rebuilds:
- Use const constructors for stateless widgets.
- Utilize
const
wherever possible to ensure widgets are only rebuilt when necessary. - Implement
shouldRebuild
inAutomaticKeepAliveClientMixin
to preserve state across rebuilds.
2. Optimize ListViews
ListView performance is critical for apps with large datasets. Improve ListView performance by:
- Using
ListView.builder
orListView.separated
to efficiently render only visible items. - Implementing
ItemBuilder
to create items on demand, reducing memory usage. - Implementing
ListView.custom
for dynamic or custom layouts.
3. Asset Optimization
Large asset files can impact app size and load times. Optimize asset usage by:
- Compressing images to reduce file size without sacrificing quality.
- Utilizing
flutter_image_compress
orflutter_native_image
for runtime image compression. - Prefetching assets with
CachedNetworkImage
orprecacheImage
to reduce loading times.
4. Managing Memory
Efficient memory management is essential for app stability and performance. Manage memory effectively by:
- Using
flutter analyze
anddart:developer
tools to identify memory leaks and performance bottlenecks. - Employing
flutter_memory_monitor
to monitor memory usage and identify optimization opportunities. - Implementing lazy loading and object pooling to minimize memory consumption.
5. Network Calls Optimization
Network calls are a common source of performance bottlenecks. Optimize network calls by:
- Using
http
package for HTTP requests anddio
for advanced features like retries and caching. - Implementing pagination and caching to reduce network usage and improve data loading times.
- Utilizing background processing and isolates for CPU-intensive tasks to prevent UI freezes.
6. Profile and Debug
Profiling and debugging are essential for identifying performance issues. Profile and debug your app by:
- Using Flutter DevTools to analyze performance metrics, including CPU, memory, and rendering.
- Utilizing Flutter Inspector to visualize widget trees and identify inefficient layouts.
- Implementing performance tests with
flutter_driver
to identify regressions and bottlenecks.
7. Code Optimization
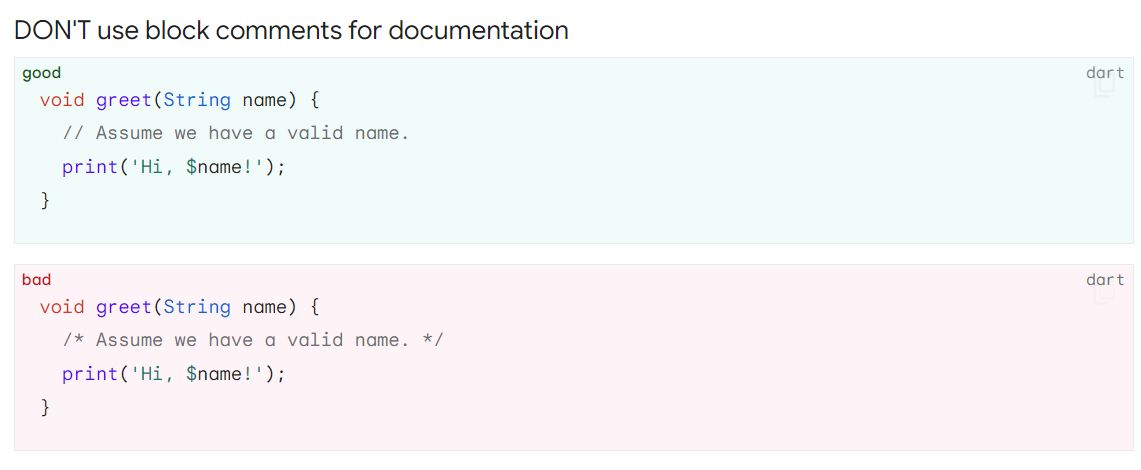
Writing efficient code can significantly impact app performance. Optimize your codebase by:
- Avoiding nested
setState
calls and unnecessary widget rebuilds. - Utilizing const constructors and final fields to minimize object creation.
- Removing unused imports and dependencies to reduce app size and startup time.
8. Platform-specific Optimization
Consider platform-specific optimizations to maximize performance on different devices:
- Utilize platform channels for native code integration and performance-critical tasks.
- Implement platform-specific optimizations for Android (e.g., ProGuard, AOT compilation) and iOS (e.g., App Thinning, Metal API).
Conclusion
Optimizing Flutter app performance is an ongoing process that requires continuous monitoring and refinement. By following these best practices and tips, you can create high-performance Flutter apps that deliver a smooth user experience across devices.