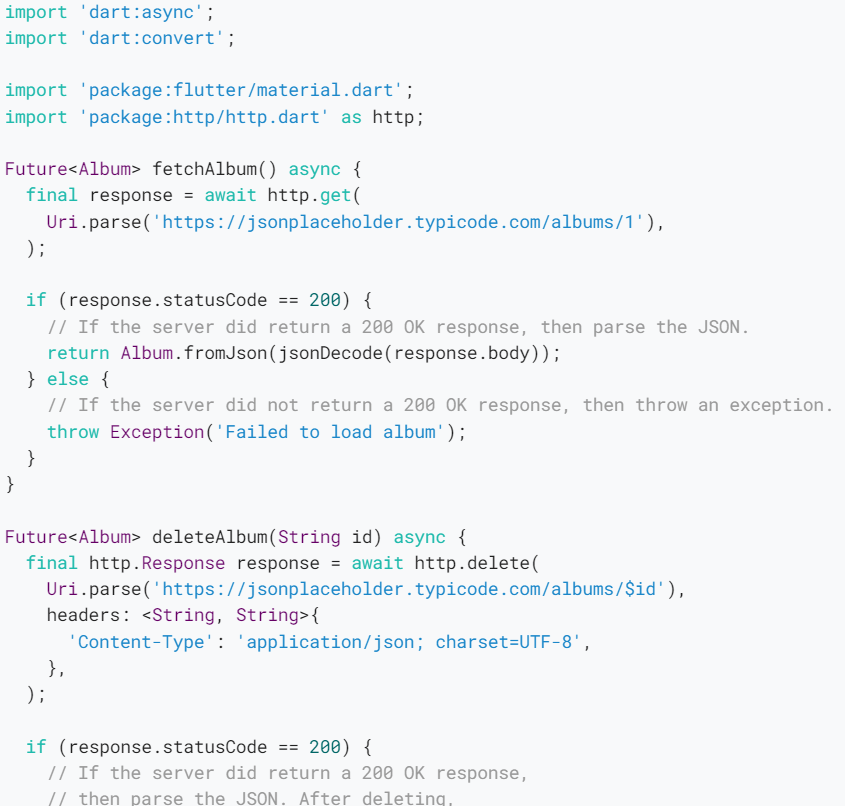
Today’s blog was about using http once again! But this time, I’m going to show you how to delete data using http on Flutter.
In contrast to my old blog post where I showed you how to fetch data using http, let’s get started!
First, you need to install the http package on Flutter to delete data on the internet. We’re going to do three operations, which are first add the package, second delete data on the server, and finally update our screen.
Adding http package
To install the http package
, add it to the pubspec.yaml
file’s dependencies section. The current version of the http package can be found at pub.dev
. This will add the following dependencies below.
dependencies:
http: <latest_version>
Don’t forget to import it.
import 'package:http/http.dart' as http;
Delete Data Using Http
Now to delete data on the server for demonstration, I am going to use the JSONPlaceholder to delete an album. Please keep in mind that the id of the album you wish to delete is required. Use anything familiar for this example, such as id = 1.
Future<http.Response> deleteAlbum(String id) async {
final http.Response response = await http.delete(
Uri.parse('https://jsonplaceholder.typicode.com/albums/$id'),
headers: <String, String>{
'Content-Type': 'application/json; charset=UTF-8',
},
);
return response;
}
Accordingly, http.delete()
returns a Future
that contains a Response
. Furthermore, Future
is a fundamental Dart class for performing async actions. A Future
object represents a possible value or error that will become available in the future, and the data obtained from a successful http call is stored in the http.Response
class. Additionally, the deleteAlbum()
method requires an id argument to identify the data to be removed from the server.
Update the Screen On Flutter
To determine whether or not the data has been removed, first retrieve it from the JSONPlaceholder
using the http.get()
method and show it on the screen. (For a comprehensive example, see the Fetch Data recipe.) You should now have a Delete Data button that, when touched, calls the deleteAlbum()
method.
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(snapshot.data?.title ?? 'Deleted'),
ElevatedButton(
child: const Text('Delete Data'),
onPressed: () {
setState(() {
_futureAlbum =
deleteAlbum(snapshot. data!.id.toString());
});
},
),
],
);
When you click the Delete Data button, the deleteAlbum()
method is invoked, and the id you supply is the id of the data you downloaded from the internet. This implies you’ll be deleting the identical stuff you downloaded from the internet.
Delete Data Using Http On Flutter Conclusion
In conclusion, I’ve shown you how to delete data using http on Flutter. To do so, you need to install the http package, add the package, delete data on the server using the http delete method, and update your screen to view the changes. The id of the album you wish to delete is required for the deleteAlbum() method to delete an album. Thank you for reading. If you found this useful, please share it with your family and friends.