For gamers, Discord is a voice and text communication platform.
Discord is used by players, streamers, and developers to talk about games, answer questions, chat while playing, and much more. There’s even a game store with critical reviews and a subscription service. For gaming communities, it’s almost a one-stop-shop.
While Discord’s APIs can be used to build a variety of things, this tutorial will focus on one specific learning outcome: how to create a Discord bot in Python.
What Is a Bot?
Discord is becoming increasingly popular. As a result, for a community to thrive and grow, automated processes such as banning inappropriate users and responding to user requests are critical.
Bot users are automated programs that look and act like users and respond to events and commands on Discord automatically. Users of Discord bots (or just bots) have nearly limitless options.
Let’s say you’re in charge of a new Discord guild and a new user joins for the first time. If you’re feeling particularly enthusiastic, you can personally reach out to that user and welcome them to your community. You could also inform them of your channels or request that they introduce themselves.
The user feels welcomed and enjoys the conversations in your guild, so they invite their friends.
Your guild’s community grows to the point where it’s no longer feasible to personally reach out to each new member, but you still want to send them something to acknowledge their arrival.
It’s possible to automatically react to a new member joining your guild using a bot. You can even control how it interacts with each new user by customizing its behavior based on context.
This is fantastic, but it’s just one example of how a bot can be beneficial. Once you learn how to make bots, there are a plethora of ways to be creative with them.
Setting Up Your Bot
To get started, first head to
And Create a New Application
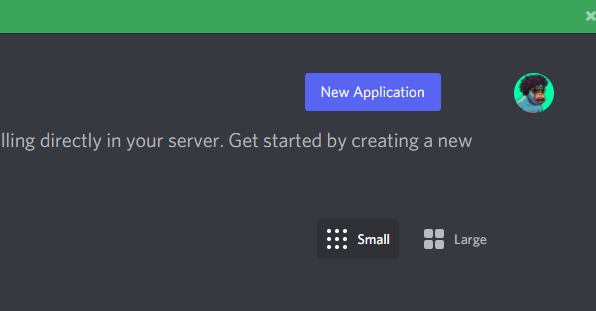
From there, head to the “Bot” tab and create a new bot.
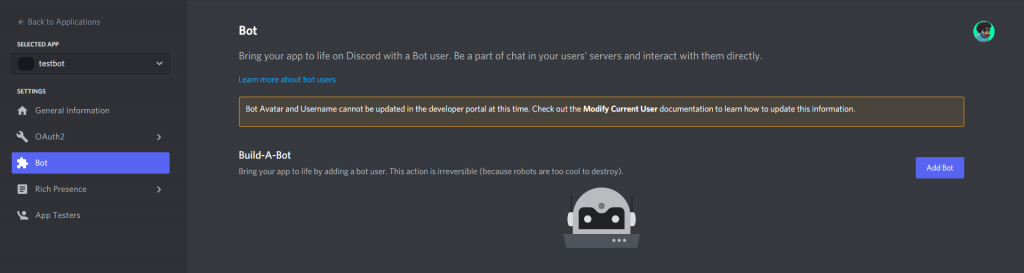
Finally, to add our bot to a server, go to the oAuth2 tab, scroll down to scopes, check bot and visit the generated URL.
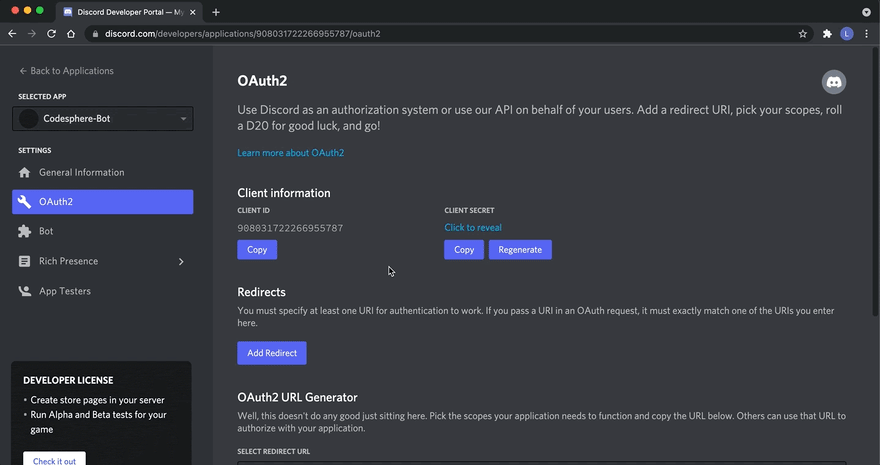
You can then select the server you want to add the bot to and you should see it on your server under offline users
Let’s Start Coding
You’ll be using discord.py because you’re learning how to make a Discord bot with Python.
discord.py is a Python library that fully implements Discord’s APIs in a Pythonic and efficient manner. This includes utilizing Python’s Async IO implementation.
To get started, use pip to install discord.py:
$ pip install -U discord.py
Now that you’ve installed discord.py, you’ll use it to create your first connection to Discord!
Establish a discord Connection
Creating a Discord connection is the first step in implementing your bot user. You do this with discord.py by creating a Client: instance.
# bot.py
import os
import discord
from dotenv import load_dotenv
load_dotenv()
TOKEN = os.getenv('DISCORD_TOKEN')
client = discord.Client()
@client.event
async def on_ready():
print(f'{client.user} has connected to Discord!')
client.run(TOKEN)
A Discord client is an object that represents a Discord connection. A client is a program that manages events, tracks states, and interacts with Discord APIs in general.
You’ve created a Client and implemented its on_ready() event handler, which handles the event when the Client has established a Discord connection and has finished preparing the data that Discord has sent, such as login state, guild, and channel data.
In other words, when the client is ready for more action, on ready() will be called (and your message will be printed). Later in this article, you’ll learn more about event handlers.
When working with secrets, such as your Discord token, it’s best to read it from an environment variable into your program. Using environment variables aids you in achieving the following goals:
- Avoid putting the secrets into source control
- Use different variables for development and production environments without changing your code
While you could export DISCORD TOKEN=your-bot-token, saving an a.env file on all machines that will be running this code is a better option. This is not only more convenient because you won’t have to export your token every time you clear your shell, but it also prevents your secrets from being stored in the history of your shell.
In the same directory as bot.py, create a file called.env:
# .env
DISCORD_TOKEN={your-bot-token}
Replace your-bot-token with your bot’s token, which you can get by returning to the Developer Portal’s Bot page and clicking Copy under the TOKEN section:
You’ll notice a library called dotenv in the bot.py code if you go back and look at it. Working with.env files is a breeze with this library. load dotenv() imports environment variables from a.env file into the environment variables of your shell.
Using pip, install dotenv:
$ pip install -U python-dotenv
Finally, client.run() uses your bot’s token to run your Client.
You can run your code now that you’ve set up bot.py and.env:
$ python bot.py
Great! Your client has established a Discord connection using your bot’s token. You’ll expand on this Client in the next section by interacting with more Discord APIs.