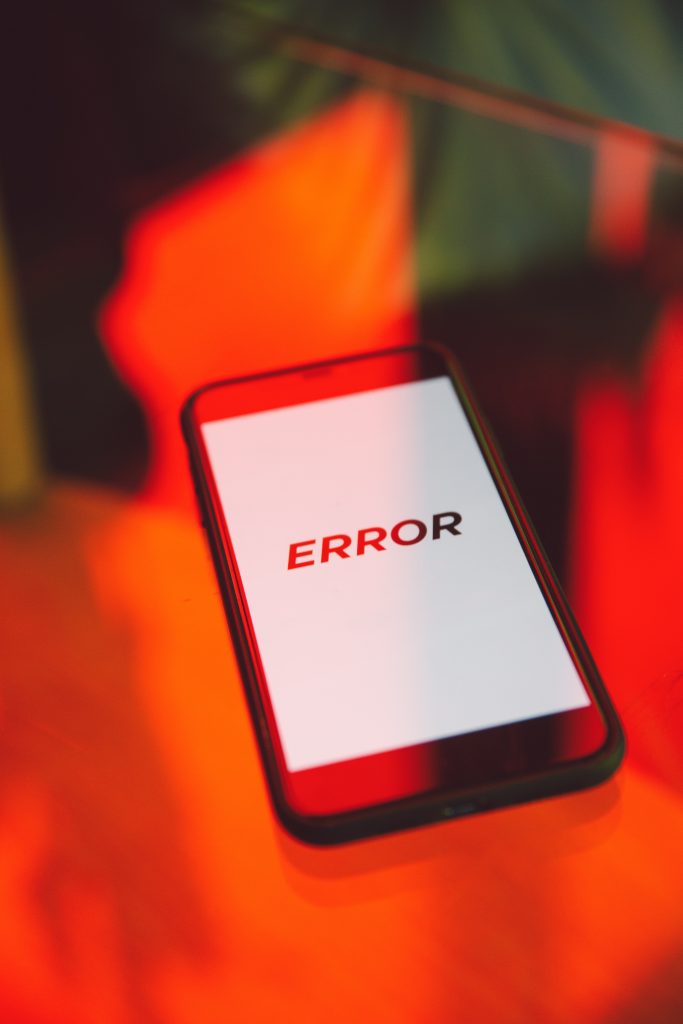
Every now and then, when developing your Flutter App, you will encounter an error, and if it was a runtime error, it may cause a problem in which your app becomes stuck on the error with no solution or alternative output. That is why today I am going to show you in this article how to handle your image errors in Flutter and prevent unslighly image asset error in the app, especially when it’s already deployed.
Error Scenario
Suppose you have an app that has a dynamic code, name, or even paths, and you can see an error might occur in this scenario. I have my app below with its source code of the widget where the image loads. It will be changed to catch the error it makes when the image loads incorrectly.
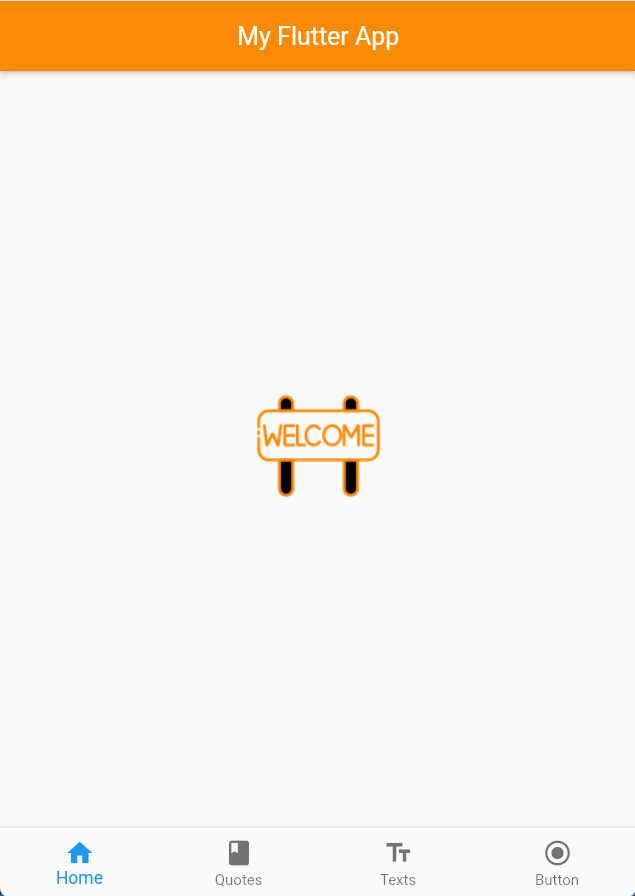
class BodyImageWidget extends StatelessWidget {
const BodyImageWidget({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Center(
child: Image.asset("images/WelcomeImage.png"),
);
}
}
What if we simply change the image name in the code above from “WelcomeImage.png” to “WelcomeImages.png”? This will cause the error shown below.
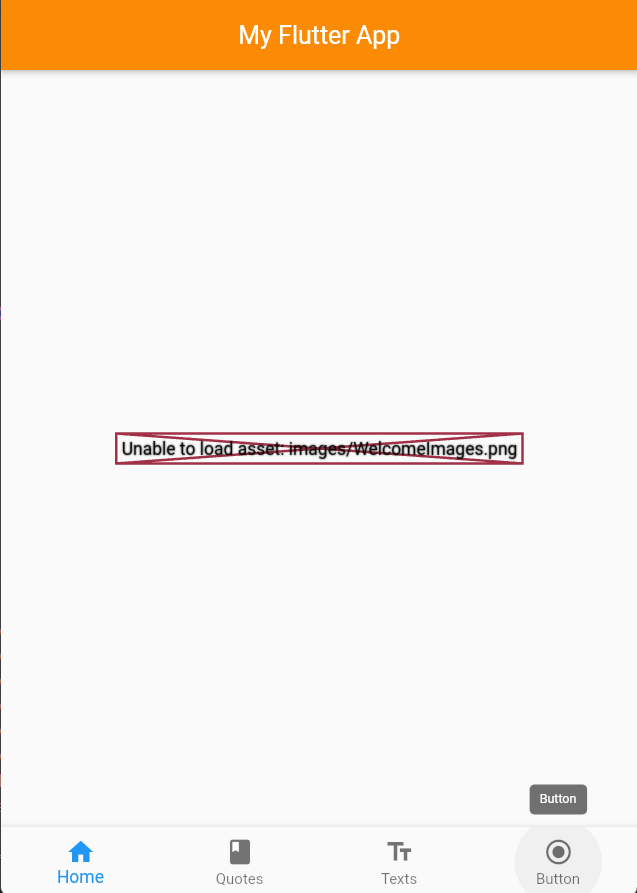
It was an unslightly image asset error. That is why I am going to introduce to you a solution.
Image ErrorBuilder Solution
The solution would be to utilize Flutter’s image argument called “errorBuilder.” where it will show an alternative error image when the first intended image didn’t load properly, which could cause an unsightly error, especially when it’s in production already. Please check the code below to see the usage of this argument.
class BodyImageWidget extends StatelessWidget {
const BodyImageWidget({
Key? key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Center(
child: Image.asset(
"images/WelcomeImages.png",
width: 90,
errorBuilder: (context, error, stackTrace) {
return Column(
children: [ Image.asset(
"images/404cloud.gif"
), const Text('There is a problem loading this image, Please check back later.')],
);
},
),
);
}
}
Result
As you can see in the result below, it’s not showing that unslightly “Unable to load asset” error. When you apply this to your own app and your IDE is Visual Studio Code, don’t forget to terminate debugging in your app and execute “flutter pub get” as the alternative image for the error won’t load and will also cause an error in simply hotrestarting the app.
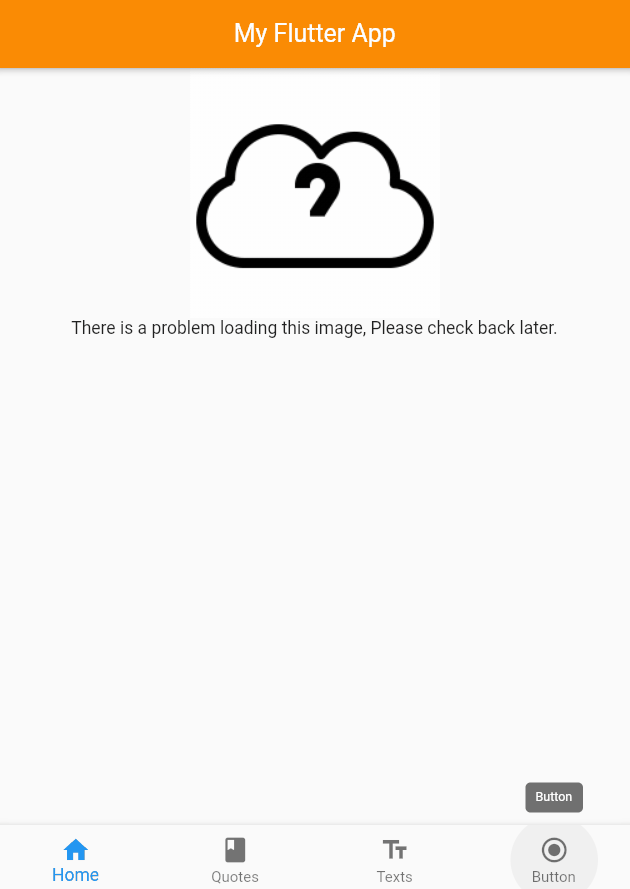
Conclusion
There’s no doubt that a simple image error can occur in your app. That is why this article discussed utilizing the Image errorBuilder argument and with some alternative images and executing the “flutter pub get” for inserting another image in the app, the “Unable to load image asset” in the app can be resolved. Thank you once again for reading and following me on this Flutter journey. Once again, farewell.
https://api.flutter.dev/flutter/widgets/Image/errorBuilder.html