There are many ways to create a circle icon Button in Flutter, but in this tutorial, we’ll cover only the following top 2 approaches:
- Using the FloatingActionButton
- Using the ElevatedButton
1. Using the FloatingActionButton (Recommended)
The FloatingActionButton is a dedicated widget to create the circular icon button.
Creating Basic Button
Steps to create a basic circle icon button using FloatingActionButton:
Step 1: Add the FloatingActionButton to your page.
Step 2: Inside FloatingActionButton, Add the child parameter and then add the actual icon inside.
FloatingActionButton(
backgroundColor: Colors.amberAccent,
onPressed: () {},
child: Icon(
Icons.train,
size: 35,
color: Colors.black,
),
),
Output:

Changing Button Size
You may want to change the default size of the FloatingActionButon to small or bigger.
To change the button size, simply replace the FloatingActionButton widget with the named constructor. i.e. FloatingActionButton.small() or FloatingActionButton.large()
FloatingActionButton.small( //<-- SEE HERE
backgroundColor: Colors.amberAccent,
onPressed: () {},
child: Icon(
Icons.train,
size: 35,
color: Colors.black,
),
),
FloatingActionButton.large( //<-- SEE HERE
backgroundColor: Colors.amberAccent,
onPressed: () {},
child: Icon(
Icons.train,
size: 35,
color: Colors.black,
),
),
Output:
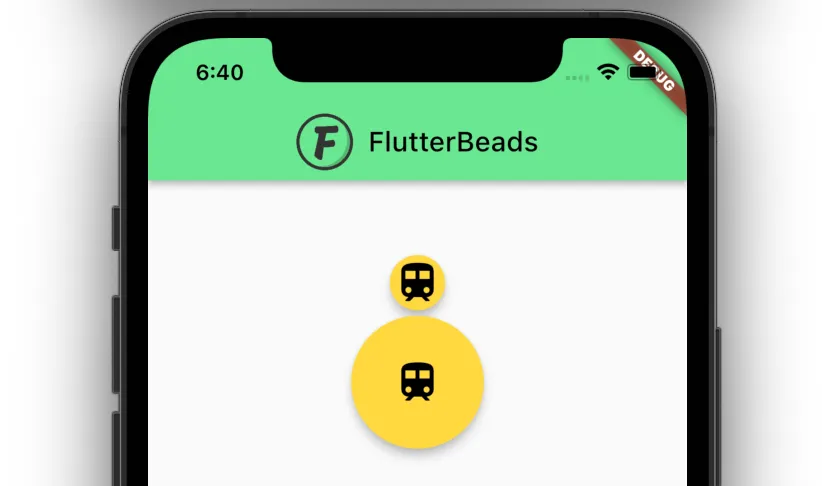
Custom Size
Sometimes you may want to have a custom size button rather than going with the predefined-sized button.
To create a circle button with custom size:
Step 1: Add the SizedBox widget to your page and give fixed height, width.
Step 2: Inside the SizedBox, add the FittedBox widget.
Step 3: Inside the FittedBox widget, add the FloatingActionButton as you would normally do.
SizedBox( //<-- SEE HERE
width: 150,
height: 150,
child: FittedBox( //<-- SEE HERE
child: FloatingActionButton( //<-- SEE HERE
backgroundColor: Colors.amberAccent,
onPressed: () {},
child: Icon(
Icons.train,
size: 35,
color: Colors.black,
),
),
),
),
Output:

2. Using the ElevatedButton
If at all the FloatingActionButton is unable to fulfill your requirement, you can use the ElevatedButton.
Creating Basic Button
Steps to create a basic circle icon button using ElevatedButton:
Step 1: Add the ElevatedButton to your page.
Step 2: Inside ElevatedButton, Add the child parameter and then add the actual icon inside.
Step 3: Inside ElevatedButton, Add the style parameter and then add the ElevatedButton.styleFrom.
Step 4: Inside the ElevatedButton.styleFrom add the shape parameter and assign the CircleBorder class.
ElevatedButton(
onPressed: () {},
child: Icon( //<-- SEE HERE
Icons.train,
color: Colors.black,
size: 54,
),
style: ElevatedButton.styleFrom(
shape: CircleBorder(), //<-- SEE HERE
padding: EdgeInsets.all(20),
),
),
Output:
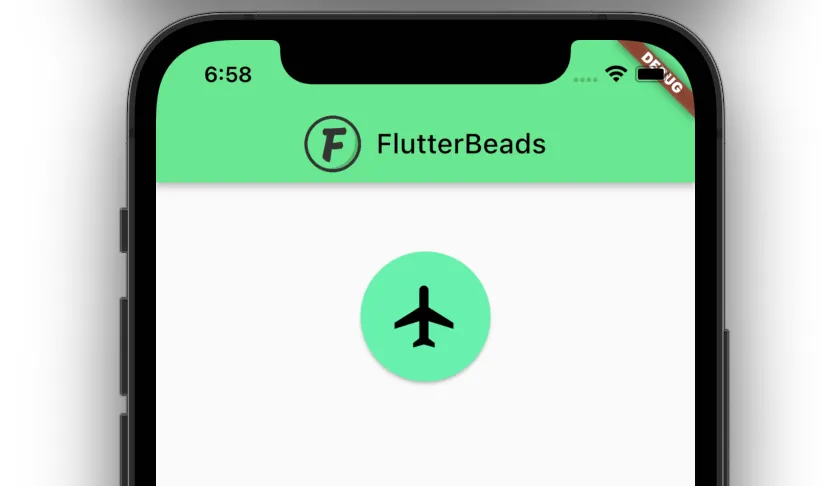