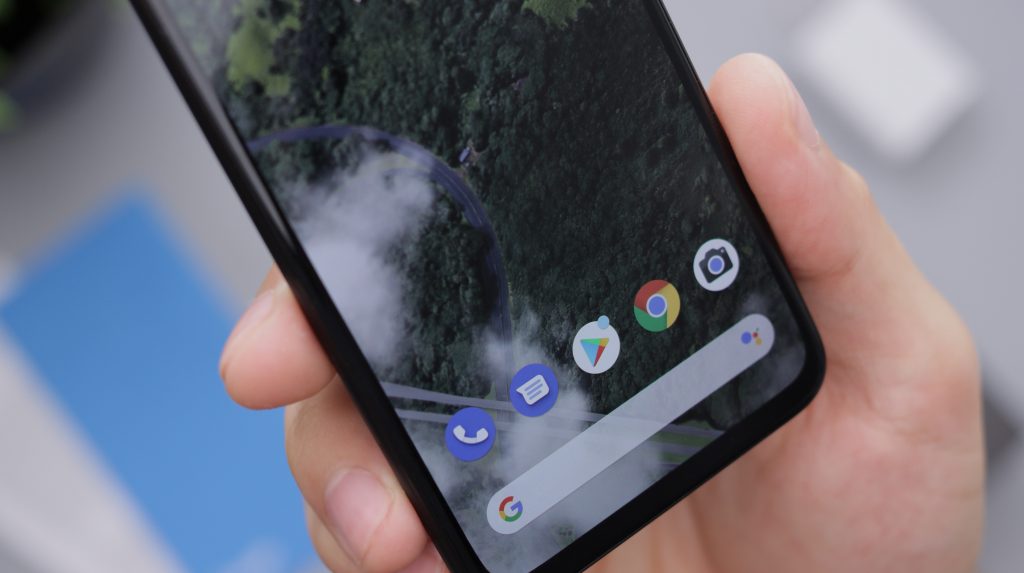
A text box (input box), text field, or text entry box is a graphical user interface control element that allows the user to enter text information to be used by a computer. On the other hand, in flutter, it’s not so different as Text fields in Flutter allow users to enter text into a UI. They typically appear in forms and dialogs. There are two types of text fields in Flutter: one is a filled text field and the other is an outlined text field. Both forms of text fields employ a container to give a clear affordance for interaction, allowing the fields to be found in layouts.
Text fields Fundamentals
Before implementing text fields in flutter, we must understand its fundamentals, which is that first it should be discoverable. In contrast, it should stand out and indicate that users can input information. Second, text field states should be clearly differentiated from one another. Third, it should be efficient as text fields should make it easy to understand the requested information and to address any errors.
Additionally, if you’re using both types of text fields, you must separate them in a user interface by area. If the text field is selected, the label text should always be visible, moving from the center to the top of the text field. Furthermore, Label text shouldn’t be truncated. Keep it short, clear, and fully visible. Don’t place error text under helper text, as their appearance will shift content.
Text Fields Implementation Code
TextFormField(
cursorColor: Theme.of(context).cursorColor,
initialValue: 'Input text',
maxLength: 20,
decoration: InputDecoration(
icon: Icon(Icons.favorite),
labelText: 'Label text',
labelStyle: TextStyle(
color: Color(0xFF6200EE),
),
helperText: 'Helper text',
suffixIcon: Icon(
Icons.check_circle,
),
enabledBorder: UnderlineInputBorder(
borderSide: BorderSide(color: Color(0xFF6200EE)),
),
),
),
Conclusion
To conclude, text fields in Flutter allow users to enter text into a UI. There are two types of text fields in Flutter—filled and outlined. Separate them in user interfaces by area to ensure they’re discoverable and easy to use. Don’t place error text under helper text, as their appearance will shift content. Finally, If you find this documentation outdated in the future, you should check the official documentation here. Thank you for reading, and please subscribe to my blog here.