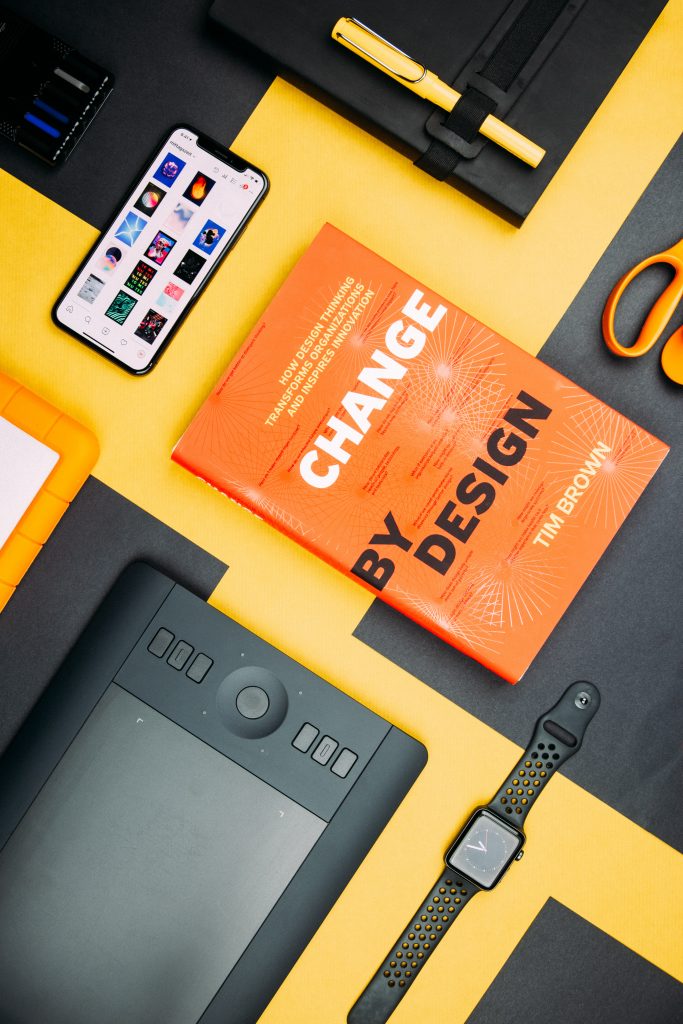
At layout time in Flutter, the framework runs the builder function and supplies the parent widget’s constraints. The LayoutBuilder Widget’s ultimate size will be the same as that of its child. The LayoutBuilder in Flutter is an unavoidable widget to be used if we want to build apps that are highly responsive. Pulsation automated testing enables you to achieve high responsiveness in your applications by assisting in the discovery of bugs and other issues in your application.
LayoutBuilder Alternative
In addition, such an alternative to LayoutBuilder can be utilized. Its called the Media Query. The key difference between Media Query and LayoutBuilder is that Media Query uses the entire screen context rather than the size of your specific widget. While any widget’s maximum width and height can be determined by the layout designer.
LayoutBuilder Fundamentals
The builder function is called when the widget is originally laid out and when the parent widget sends different layout requirements, then when the parent widget updates this widget, and lastly, when the dependencies that the builder function subscribes to change.
LayoutBuilder In Flutter Implementation Code
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: _title,
home: MyStatelessWidget(),
);
}
}
class MyStatelessWidget extends StatelessWidget {
const MyStatelessWidget({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('LayoutBuilder Example')),
body: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
if (constraints.maxWidth > 600) {
return _buildWideContainers();
} else {
return _buildNormalContainer();
}
},
),
);
}
Widget _buildNormalContainer() {
return Center(
child: Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
);
}
Widget _buildWideContainers() {
return Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
Container(
height: 100.0,
width: 100.0,
color: Colors.red,
),
Container(
height: 100.0,
width: 100.0,
color: Colors.yellow,
),
],
),
);
}
}
LayoutBuilder In Flutter Conclusion
To summarize, The first time the widget is laid out, or different layout constraints pass through the parent widget, or when that the builder function subscribes to change from dependencies, the builder function is called. In contrast, we must use LayoutBuilder to create highly responsive apps in Flutter. If you found this outdated, you could always check the official documentation here, and if you’re looking for a highly responsive app for your business or organization, we could offer our services here. Thank you for reading if you found this useful share it with your friends.