In this tutorial, you will learn how to create a simple calculator using PHP. You will learn how to take user input, perform arithmetic operations, and display the results. This tutorial is suitable for beginner PHP developers who want to improve their skills by building a functional calculator application. By the end of this tutorial, you will have a better understanding of how PHP handles user inputs and basic arithmetic operations.
HTML
Create the HTML structure for the calculator: Use HTML to create a form with input fields for the user to enter numbers and select operations.
Here’s the HTML structure for the calculator form:
<main>
<section>
<form method="post">
<input type="text" name="num1" placeholder="Enter first number">
<br><br>
<input type="text" name="num2" placeholder="Enter second number">
<br><br>
<select name="operator">
<option value="Addition">Addition</option>
<option value="Subtraction">Subtraction</option>
<option value="Multiplication">Multiplication</option>
<option value="Division">Division</option>
</select>
<br><br>
<input type="submit" name="count" value="Calculate">
<br><br>
</form>
</section>
</main>
These lines of HTML code define two input fields for the user to enter the numbers that they want to perform the calculation with.
<input type="text" name="num1" placeholder="Enter first number">
<input type="text" name="num2" placeholder="Enter second number">
This line of HTML code creates a dropdown list to select the mathematical operation the user wants to perform on the numbers entered.
<select name="operator">
<option value="Addition">Addition</option>
<option value="Subtraction">Subtraction</option>
<option value="Multiplication">Multiplication</option>
<option value="Division">Division</option>
</select>
This line of HTML code creates a submit button to send the form data to the server.
<input type="submit" name="count" value="Calculate">
CSS
CSS (Cascading Style Sheets) is a style sheet language used for describing the look and formatting of a document written in HTML. In this simple calculator program, CSS can be used to enhance the appearance of the HTML elements and make the user interface more visually appealing. CSS can be used to define the styles for the form elements, such as the text boxes, dropdown list, and submit button, as well as the font, colors, and background. By using CSS, you can make the calculator look professional and easy to use.
Here’s a simple CSS design for the calculator form:
<style>
body {
border: border-box;
padding: 0;
margin: 0;
font-family: 'Poppins', sans-serif;
}
main {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
form {
width: 500px;
margin: 50px auto;
text-align: center;
padding: 20px;
border: 1px solid #ccc;
box-shadow: 2px 2px 5px #ccc;
}
input[type="text"], select {
width: 100%;
padding: 10px;
margin: 10px 0;
box-sizing: border-box;
border: 1px solid #ccc;
border-radius: 4px;
}
input[type="submit"] {
width: 100%;
padding: 10px;
margin: 10px 0;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #3e8e41;
}
input[type="text"] {
background-color: #f2f2f2;
}
</style>
This CSS design sets a width for the form and centers it on the page. It styles the input fields and the submit button with a width, padding, margin, border, border-radius, and background color. It also adds hover effect for the submit button. The input field for the result has a light gray background color. This design adds some basic styling to the calculator form to make it visually appealing to the user.
PHP
The PHP code in this simple calculator program performs the calculation based on the user inputs. When the user enters two numbers and selects a mathematical operation from the dropdown list, the form data is sent to the server. The PHP code then retrieves the user inputs and performs the calculation based on the selected operation.
Here’s an PHP code with validation:
<?php
if(isset($_POST['count'])) {
$num1 = $_POST['num1'];
$num2 = $_POST['num2'];
$operator = $_POST['operator'];
// validate user inputs
if (!is_numeric($num1) || !is_numeric($num2)) {
$error = "Both inputs must be numeric values.";
} else {
switch($operator) {
case 'Addition':
$result = $num1 + $num2;
break;
case 'Subtraction':
$result = $num1 - $num2;
break;
case 'Multiplication':
$result = $num1 * $num2;
break;
case 'Division':
// avoid division by zero
if ($num2 == 0) {
$error = "Cannot divide by zero.";
} else {
$result = $num1 / $num2;
}
break;
}
}
}
?>
if(isset($_POST['count']))
: This line checks if the form has been submitted.$_POST['count']
refers to the value of the submit button named “count”. If the form has been submitted, the code inside the if statement will be executed.$num1 = $_POST['num1'];
: This line retrieves the value of the first number input field and stores it in the$num1
variable.$num2 = $_POST['num2'];
: This line retrieves the value of the second number input field and stores it in the$num2
variable.$operator = $_POST['operator'];
: This line retrieves the selected mathematical operation from the dropdown list and stores it in the$operator
variable.if (!is_numeric($num1) || !is_numeric($num2))
: This line validates the user inputs to ensure that both inputs are numeric values. Theis_numeric
function returnsTRUE
if the value passed to it is a number or a string representing a number, andFALSE
otherwise. If either$num1
or$num2
is not a numeric value, the error message “Both inputs must be numeric values.” is assigned to the$error
variable.switch($operator)
: This line starts a switch statement that performs different calculations based on the selected mathematical operation.case 'Addition':
: If the selected mathematical operation is “Addition”, the calculation$result = $num1 + $num2;
is performed, and the result is stored in the$result
variable.case 'Subtraction':
: If the selected mathematical operation is “Subtraction”, the calculation$result = $num1 - $num2;
is performed, and the result is stored in the$result
variable.case 'Multiplication':
: If the selected mathematical operation is “Multiplication”, the calculation$result = $num1 * $num2;
is performed, and the result is stored in the$result
variable.case 'Division':
: If the selected mathematical operation is “Division”, the following code block checks if$num2
is equal to zero. If it is, the error message “Cannot divide by zero.” is assigned to the$error
variable. If$num2
is not equal to zero, the calculation$result = $num1 / $num2;
is performed, and the result is stored in the$result
variable.
Add this code to the HTML Structure for the validation and results.
<?php if (isset($result)) { ?>
<input type="text" value="<?php echo $result; ?>" readonly>
<?php } ?>
<?php if (isset($error)) { ?>
<p style="color:red;"><?php echo $error; ?></p>
<?php } ?>
Here’s the complete structure of the code.
<!--Simple Calculator using PHP-->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Calculator</title>
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,700;0,800;0,900;1,100;1,200;1,300;1,400;1,500;1,600;1,700;1,800;1,900&display=swap" rel="stylesheet">
</head>
<style>
body {
border: border-box;
padding: 0;
margin: 0;
font-family: 'Poppins', sans-serif;
}
main {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
}
form {
width: 500px;
margin: 50px auto;
text-align: center;
padding: 20px;
border: 1px solid #ccc;
box-shadow: 2px 2px 5px #ccc;
}
input[type="text"], select {
width: 100%;
padding: 10px;
margin: 10px 0;
box-sizing: border-box;
border: 1px solid #ccc;
border-radius: 4px;
}
input[type="submit"] {
width: 100%;
padding: 10px;
margin: 10px 0;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #3e8e41;
}
input[type="text"] {
background-color: #f2f2f2;
}
</style>
<body>
<?php
if(isset($_POST['count'])) {
$num1 = $_POST['num1'];
$num2 = $_POST['num2'];
$operator = $_POST['operator'];
// validate user inputs
if (!is_numeric($num1) || !is_numeric($num2)) {
$error = "Both inputs must be numeric values.";
} else {
switch($operator) {
case 'Addition':
$result = $num1 + $num2;
break;
case 'Subtraction':
$result = $num1 - $num2;
break;
case 'Multiplication':
$result = $num1 * $num2;
break;
case 'Division':
// avoid division by zero
if ($num2 == 0) {
$error = "Cannot divide by zero.";
} else {
$result = $num1 / $num2;
}
break;
}
}
}
?>
<main>
<section>
<form method="post">
<input type="text" name="num1" placeholder="Enter first number">
<br><br>
<input type="text" name="num2" placeholder="Enter second number">
<br><br>
<select name="operator">
<option value="Addition">Addition</option>
<option value="Subtraction">Subtraction</option>
<option value="Multiplication">Multiplication</option>
<option value="Division">Division</option>
</select>
<br><br>
<input type="submit" name="count" value="Calculate">
<br><br>
<?php if (isset($result)) { ?>
<input type="text" value="<?php echo $result; ?>" readonly>
<?php } ?>
<?php if (isset($error)) { ?>
<p style="color:red;"><?php echo $error; ?></p>
<?php } ?>
</form>
</section>
</main>
</body>
</html>
And here’s the results:
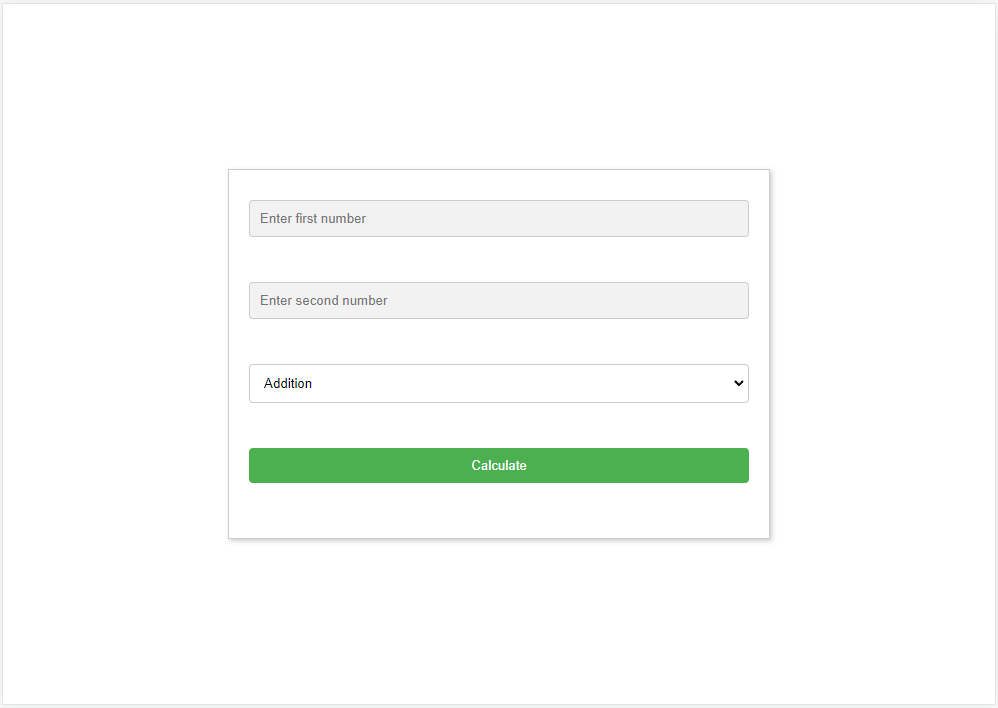
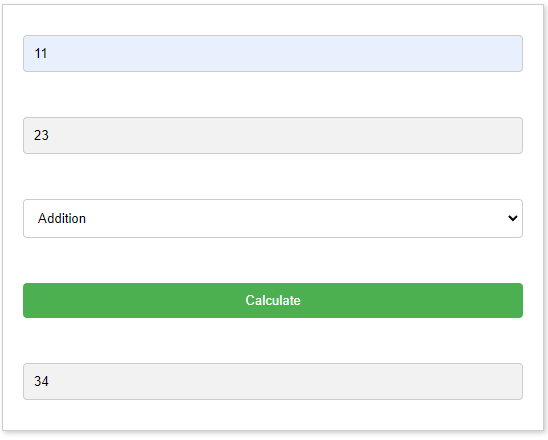
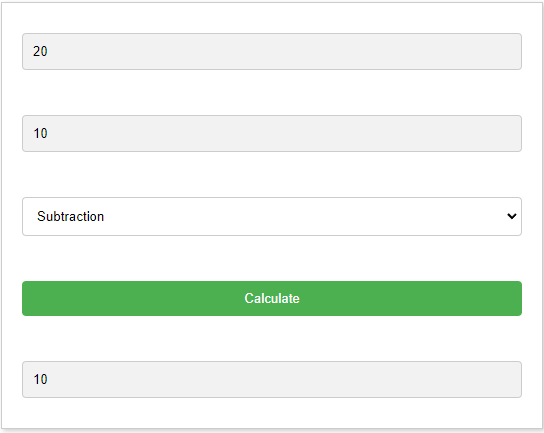
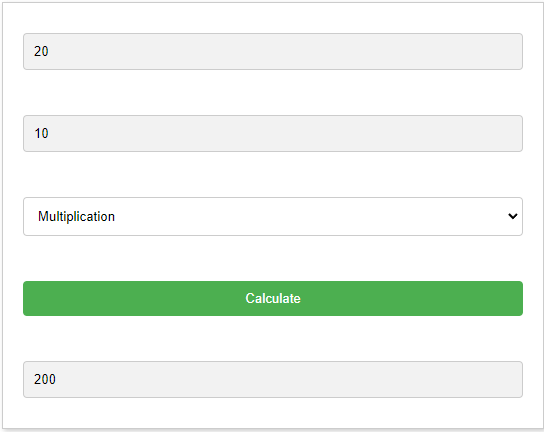
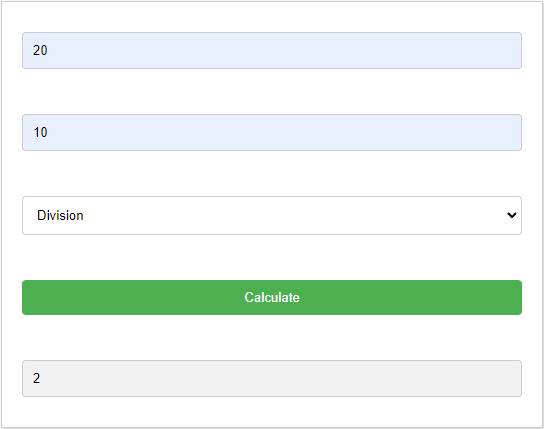
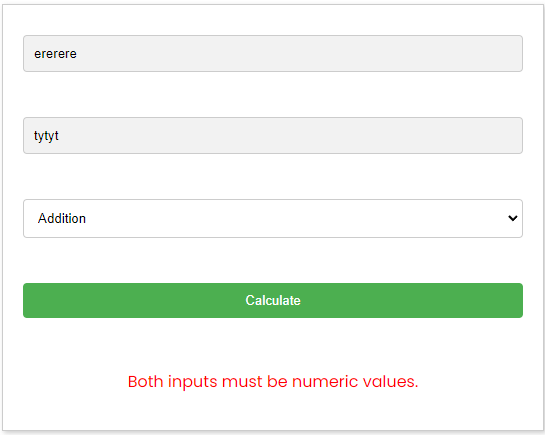
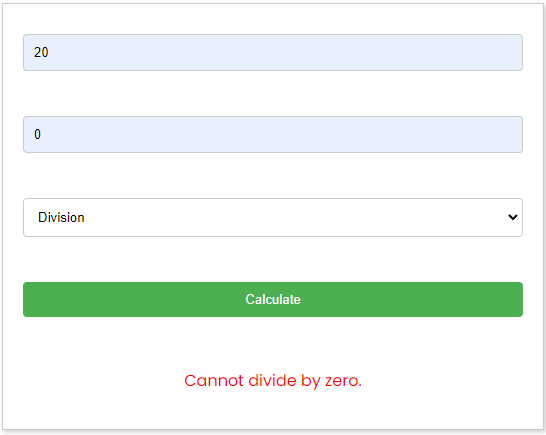