Introduction
Arithmetic Operators in Python can perform addition, subtraction, multiplication, division, exponentiation, and modulus operators. In this blog, I am going to teach you how to do 4 basic arithmetic operations with methods in Python.
Creating Functions
Before we go into the meat and potatoes if this topic, let us first initialize our functions. In Python, functions are blocks of code that only run when it is called. Inside this functions, we can pass data, also known as parameters.
Let us examine the code below:
def addition(num1, num2):
return num1 + num2
Functions are initialized with the keyword “def”. And if you hover over the keyword, it shows you a more detailed explanation.
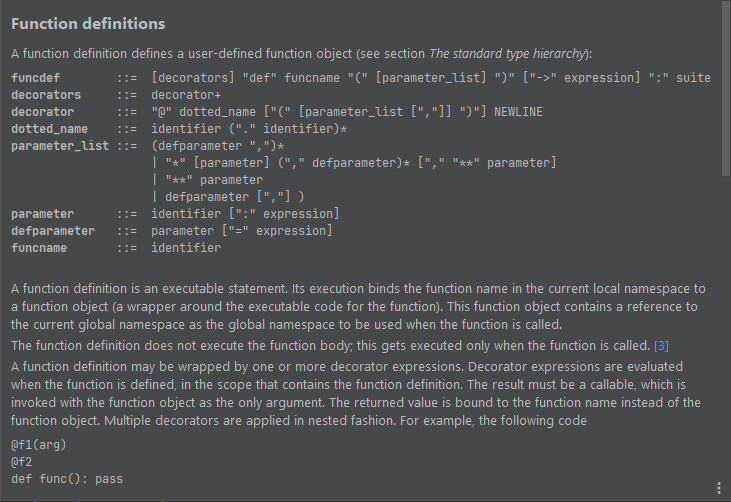
The function is named addition(), has two arguments (num1 and num2) and inside the function is a return statement with an arithmetic operation. What the return statement does is return the data back to the caller.
Now that the addition function has been created, we will now create the functions for our remaining arithmetic operations: subtraction, multiplication, division.
def subtraction(num1, num2):
if num2 > num1:
return num2 - num1
else:
return num1 - num2
def multiplication(num1, num2):
return num1 * num2
def division(num1, num2):
if num2 > num1:
return float(num2 / num1)
else:
return float(num1 / num2)
Now that our functions have been created, let us now go to creating our menu.
Creating a menu
First thing we’re going to do is make a menu. This makes it so that the user can have a choice on which arithmetic operation they are going to use. Let us start with this code below:
print("Basic Arithmetic with Methods In Python")
print("""Enter operation:
A. Addition
B. Subtraction
C. Multiplication
D. Division
E. Quit
""")
ch = input("Enter choice: ")
The first and second line prints texts for the user to see. It shows the title of the program “Basic Arithmetic with Methods In Python”. The second line lists all the possible choices of arithmetic operations that our program can perform for them. The image below shows what it looks like:
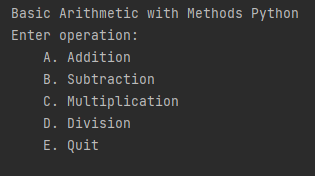
Next, we need to make our program take their input.
ch = input("Enter choice: ")
We made a variable named “ch” (short for choice). This is where we are going to be storing the user input.
expanding our menu
Since we are going to have multiple choices, we have to check if the user input matches one of them. For that, we are going to be using conditional statements.
if ch.upper() == 'A':
while ValueError:
try:
inp1 = int(input("Enter first number: "))
break
except ValueError:
print("Please enter a number")
while ValueError:
try:
inp2 = int(input("Enter second number: "))
break
except ValueError:
print("Please enter a number")
print(addition(inp1, inp2))
The first line checks if the user input is equal to ‘A’. The upper method turns all user input into capital letters. This is shorter than having to make an or condition in our line. If the user input is equal to ‘A’, the program enters into a while loop. The while loop executes a set of statements as long as the condition is true. In this case, lines 2-7 asks the user for the input to the first number. The ValueError exception occurs when a function receives an argument of the correct data type but an inappropriate value. In our code, if the user types a non-integer, it prints out “Please enter a number”. This is possible thanks to the try-except block, which lets us test a block of our code for errors.
Once the condition for the while loop has been broken, the loop now breaks due to the break statement. It now goes into the second while loop. Lines 8-13 asks for the input to the second number. Once both while loops have been broken, it now prints the sum of the two numbers in line 14.
print(addition(inp1, inp2))
What this line of code does is call the addition function and pass the arguments inp1 and inp2, which resulted in the function performing this:
return num1 + num2 # returns sum
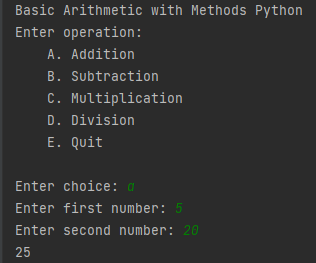
Let us now do the rest of the remaining operations.
elif ch.upper() == 'B':
while ValueError:
try:
inp1 = int(input("Enter first number: "))
break
except ValueError:
print("Please enter a number")
while ValueError:
try:
inp2 = int(input("Enter second number: "))
break
except ValueError:
print("Please enter a number")
print(subtraction(inp1, inp2))
elif ch.upper() == 'C':
while ValueError:
try:
inp1 = int(input("Enter first number: "))
break
except ValueError:
print("Please enter a number")
while ValueError:
try:
inp2 = int(input("Enter second number: "))
break
except ValueError:
print("Please enter a number")
print(multiplication(inp1, inp2))
elif ch.upper() == 'D':
while ValueError:
try:
inp1 = int(input("Enter first number: "))
break
except ValueError:
print("Please enter a number")
while ValueError:
try:
inp2 = int(input("Enter second number: "))
break
except ValueError:
print("Please enter a number")
print(division(inp1, inp2))
elif ch.upper() == 'E':
break
If the user has not inputted ‘A’ then it will go into the remaining elif statements. In python, elif statements are used to check another condition if the if statement wasn’t true. ‘B’ calls the subtraction function. ‘C’ calls the multiplication function. ‘D’ calls the division function. ‘E’ breaks the loop and ends the program.
Note: You can put as many elif statements as you want.
Figure 4. Result of Subtraction, Multiplication, and Division
else statement
To end the if-else loop properly, we close it with the else statement. What the else statement does is catch anything that wasn’t caught by preceding conditions.
else:
print("Please enter a valid choice")
Even if the user enters something by mistake, the program will not produce an error. Instead, it will go to the else statement and print out “Please enter a valid choice“.
Conclusion
Python is a fun programming language. It is very easy to learn thanks to the simple syntax that it has. Hope that all of you reading this got interested in learning Python. Who knows? Maybe in the future, you can guys can be senior developers!