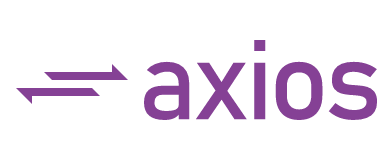
Axios is a promise-based HTTP client for the browser and Node.js. Axios makes it easy to send asynchronous HTTP requests to REST endpoints and perform CRUD operations. It can be used in plain JavaScript or with a library such as Vue or React.
The HTTP protocol is the most common way for frontend programs to communicate with a server. Maybe you are familiar with Fetch API and XMLHttpRequest interface. If you are using a Javascript library, you may also be familiar with jquery’s $.ajax() function
Advantages of using Axios
- Request and response interception
- Streamlined error handling
- Protection against XSRF
- Support for upload progress
- Response timeout
- The ability to cancel requests
- Support for older browsers
- Automatic JSON data transformation
Difference of Axios and Fetch
Axios has url in request object.
Axios is a stand-alone third party package that can be easily installed.
Axios uses the data property.
Axios’ data contains the object.
Axios request is ok when status is 200 and statusText is ‘OK’.
Axios performs automatic transforms of JSON data.
Axios has built-in support for download progress.
Axios has wide browser support.
Fetch has no url in request object.
Fetch is built into most modern browsers; no installation is required as such.
Fetch uses the body property.
Fetch’s body has to be stringified.
Fetch request is ok when response object contains the ok property.
Fetch is a two-step process when handling JSON data- first, to make the actual request; second, to call the .json() method on the response.
Fetch does not support upload progress.
Fetch only supports Chrome 42+, Firefox 39+, Edge 14+, and Safari 10.1+ (This is known as Backward Compatibility).
Installation
nmp – npm install axios
Boswer – bower install axios
CDN – <script src="https://unpkg.com/axios/dist/axios.min.js"></script>
Shorthand methods for Axios HTTP requests
axios.get(url[, config])
axios.delete(url[, config])
axios.head(url[, config])
axios.options(url[, config])
axios.post(url[, data[, config]])
axios.put(url[, data[, config]])
axios.patch(url[, data[, config]])
axios.get()
axios.get(‘https://ghibliapi.herokuapp.com/films’)
.then((response) => {
console.log(response.data);
console.log(response.status);
console.log(response.statusText);
console.log(response.headers);
});
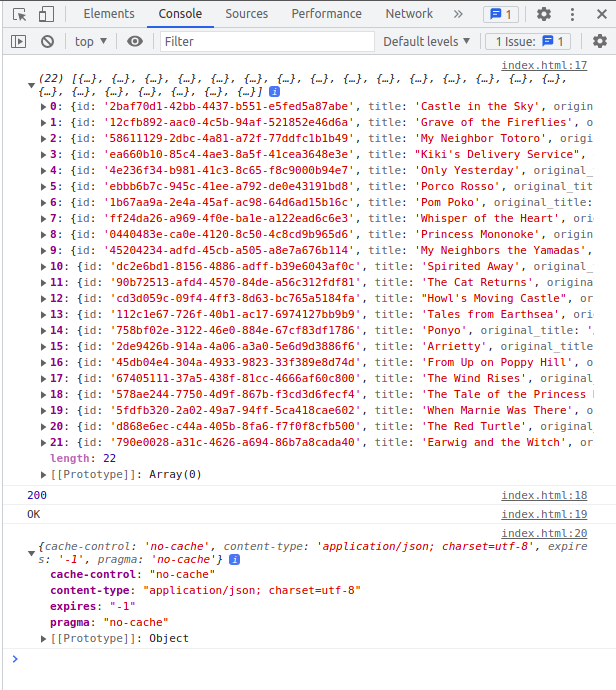
axios.post()
axios.post(‘/login’, {
firstName: ‘Finn’,
lastName: ‘Williams’
})
.then((response) => {
console.log(response);
}, (error) => {
console.log(error);
});
For post requests, Once an HTTP POST request is made, Axios returns a promise that is either fulfilled or rejected, depending on the response from the backend service. If the promise is fulfilled, the first argument of then() will be called; if the promise is rejected, the second argument will be called
Axios is popular among developers for a reason: it’s packed with helpful features. We’ve looked at some main features of Axios. However, there are still a lot of areas of Axios that we haven’t covered. Check out the Axios GitHub page for more information.
Reference
https://github.com/axios/axios
https://www.geeksforgeeks.org/difference-between-fetch-and-axios-js-for-making-http-requests/#:~:text=Axios%20has%20built%2Din%20support,is%20known%20as%20Backward%20Compatibility).